Understanding JWT: From Basics to Advanced Security
Discover how JSON Web Tokens (JWT) revolutionize web authentication. This guide covers everything from basic concepts to advanced security measures, helping you implement secure, scalable authentication in modern applications.
Introduction: The Evolution of Web Authentication
Early days of the web, authentication was relatively simple - users would log in, and servers would store their session information. However, as applications grew more complex and moved toward distributed systems and microservices, this traditional approach faced scalability challenges. JSON Web Tokens (JWTs), a revolutionary solution that changed how we handle authentication and authorization in modern web applications.
JWTs represent a paradigm shift in web security. Instead of the server keeping track of who's logged in, it issues a secure, self-contained token that holds all necessary information about the user. Think of it like a digital passport - once issued by a trusted authority, it can be verified anywhere without needing to check back with the issuing country. This approach has become increasingly important in our interconnected world of cloud services, mobile applications, and API-driven architectures.
Part 1: JWT Fundamentals
What is a JWT?
A JSON Web Token is a compact, self-contained way to securely transmit information between parties as a JSON object. Think of it as a digital passport that proves your identity and permissions in the web world. Each JWT contains three essential parts:
- Header: The metadata section that describes the token's type and the signing algorithm used
- Payload: The actual data (called "claims") about the user and additional metadata
- Signature: A verification seal that ensures the token hasn't been tampered with
When these three parts are combined, you get something that looks like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
The Anatomy of a JWT: Understanding the Structure
Before diving into how JWTs work, let's examine their structure in detail. A JWT consists of three distinct parts, each encoded in Base64URL format and separated by dots:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
1. Header (The Metadata)
The header contains information about how the token itself is constructed. It typically includes:
{
"alg": "HS256", // The algorithm used for signing
"typ": "JWT" // The type of token
}
2. Payload (The Claims)
The payload carries the actual information about the user and token metadata. It contains claims, which are statements about an entity (typically the user) and additional metadata.
There are three types of claims:
- Public Claims: Custom claims registered in the IANA JSON Web Token Registry
- Private Claims: Custom claims created for sharing information between parties
- Registered Claims: Predefined claims like:
{
"iss": "auth.service.com", // Issuer
"sub": "user123", // Subject
"exp": 1516239022, // Expiration Time
"iat": 1516232822 // Issued At
}
3. Signature (The Verification Seal)
The signature is created by taking the encoded header, encoded payload, and a secret key, then applying the algorithm specified in the header. For example, with HMAC SHA256:
HMACSHA256(
base64UrlEncode(header) + "." +
base64UrlEncode(payload),
secret
)
This signature ensures that the token hasn't been modified in transit and verifies its authenticity.
How Does JWT Work in Practice?
The journey of a JWT follows a simple flow:
- Authentication: When you log in successfully, the server creates a JWT containing information about your identity and permissions
- Token Storage: Your application receives and stores this token
- Authorization: For subsequent requests, your application includes this token in the header
- Verification: The server checks the token's signature and grants access if it's valid
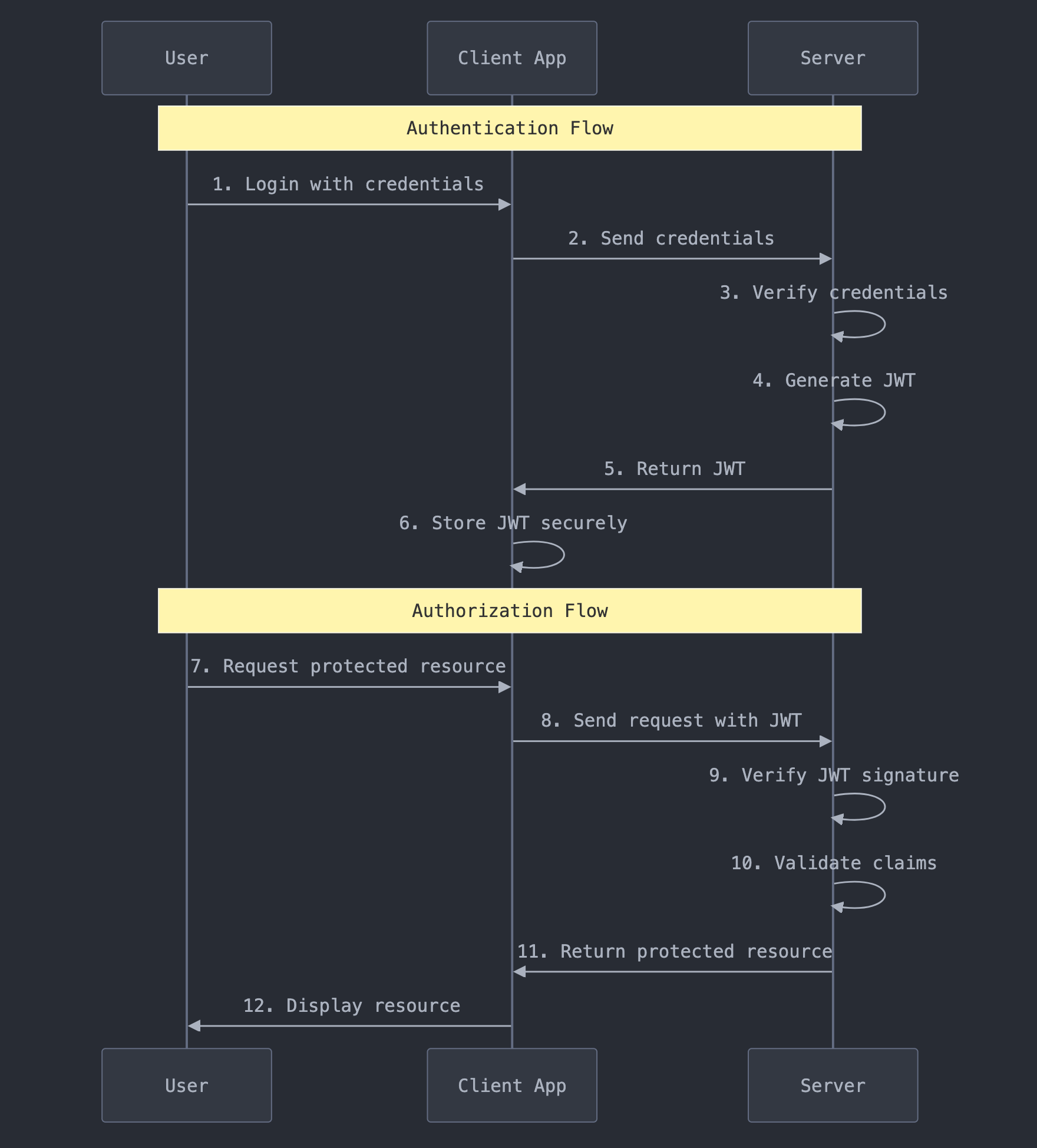
This process eliminates the need for the server to store session information, making JWTs perfect for modern, scalable applications.
Part 2: The Security Challenge
Common JWT Vulnerabilities
While JWTs are powerful, they're not immune to security issues. Here are the key vulnerabilities to watch out for:
Signature Verification Flaws
The signature is like a tamper-evident seal on a package. If the verification process is flawed or the secret key is weak, attackers can modify the token's contents without detection. This is particularly dangerous because it could allow unauthorized access to protected resources.
Algorithm Confusion Attacks
JWTs support multiple signing algorithms, including a dangerous "none" algorithm. If your server isn't configured properly, an attacker might trick it into accepting an unsigned token, effectively bypassing security checks altogether.
Client-Side Storage Risks
Many applications store JWTs in browser storage (localStorage or sessionStorage), making them vulnerable to Cross-Site Scripting (XSS) attacks. Think of this like keeping your house key in an obvious place - if someone gains access to your browser's JavaScript environment, they could steal your token.
The Revocation Challenge
Unlike traditional session tokens, JWTs don't have a built-in way to be invalidated before they expire. This means if a token is compromised, it remains valid until its expiration time, potentially giving attackers a window of opportunity.
Part 3: Building a Secure JWT Implementation
Essential Security Practices
Let's explore how to implement JWTs securely:
1. Secure Communication
Always transmit JWTs over HTTPS. This encrypts the token during transmission, preventing eavesdropping attacks. It's like sending sensitive documents through a secure courier service instead of regular mail.
2. Smart Token Storage
Instead of using browser storage, use HttpOnly cookies with the Secure flag enabled. This approach prevents JavaScript access to the token while ensuring it's only transmitted over secure connections. The cookie settings might look like this:
Set-Cookie: access_token=<token>;
HttpOnly;
Secure;
SameSite=Strict;
Path=/api
3. Token Lifecycle Management
Implement a robust token lifecycle strategy:
- Set short expiration times for access tokens (15-30 minutes)
- Use refresh tokens for obtaining new access tokens
- Maintain a token blacklist for revoked tokens
- Implement token rotation to regularly replace active tokens
4. Payload Security
Be mindful of what you include in your token payload:
- Never store sensitive data like passwords or credit card numbers
- Minimize the payload size to reduce overhead
- Consider encrypting certain claims if they contain sensitive information
Advanced Security Measures
Going beyond the basics:
1. Fingerprinting
Include device or context information in the token to detect potential token theft:
{
"sub": "1234567890",
"fingerprint": "device_id|user_agent|ip_hash",
"exp": 1516239022
}
2. Audience Validation
Specify and validate the intended recipient of the token to prevent token reuse across different services:
{
"aud": "https://api.myservice.com",
"sub": "1234567890",
"exp": 1516239022
}
Part 4: The Future of JWT Security
Emerging Security Enhancements
The security landscape is constantly evolving, and JWT security is no exception. Here are some promising developments:
1. Quantum-Resistant Algorithms
As quantum computing advances, new signing algorithms resistant to quantum attacks are being developed. These will ensure JWTs remain secure even in a post-quantum world.
2. Dynamic Validation
Future implementations might include real-time validation systems that can instantly revoke tokens based on security events or user behavior changes.
3. Enhanced Standards
The JWT standard continues to evolve with new security features and best practices. Stay updated with the latest JWT specifications and security recommendations from the IETF.
Conclusion
JWTs represent a powerful solution for modern web authentication and authorization, but their security requires careful consideration and implementation. By understanding both the fundamentals and security challenges, and by implementing robust security measures, you can harness the benefits of JWTs while maintaining strong security.
Learn on JWT, OAuth, OIDC, and SAML: https://guptadeepak.com/demystifying-jwt-oauth-oidc-and-saml-a-technical-guide/
Quick Reference: JWT Security Checklist
✓ Use strong, unique signing keys
✓ Implement proper algorithm validation
✓ Store tokens securely (HttpOnly cookies)
✓ Set appropriate token expiration
✓ Implement token rotation
✓ Validate token claims thoroughly
✓ Monitor for security updates
✓ Use HTTPS exclusively
✓ Implement proper error handling
✓ Regular security audits