Secure Coding Practices Guide: Principles, Vulnerabilities, and Verification
Discover how proper secure coding practices can prevent costly data breaches and vulnerabilities. This comprehensive guide covers essential security principles, OWASP Top 10 mitigations, and language-specific techniques that every developer needs to implement in their SDLC.
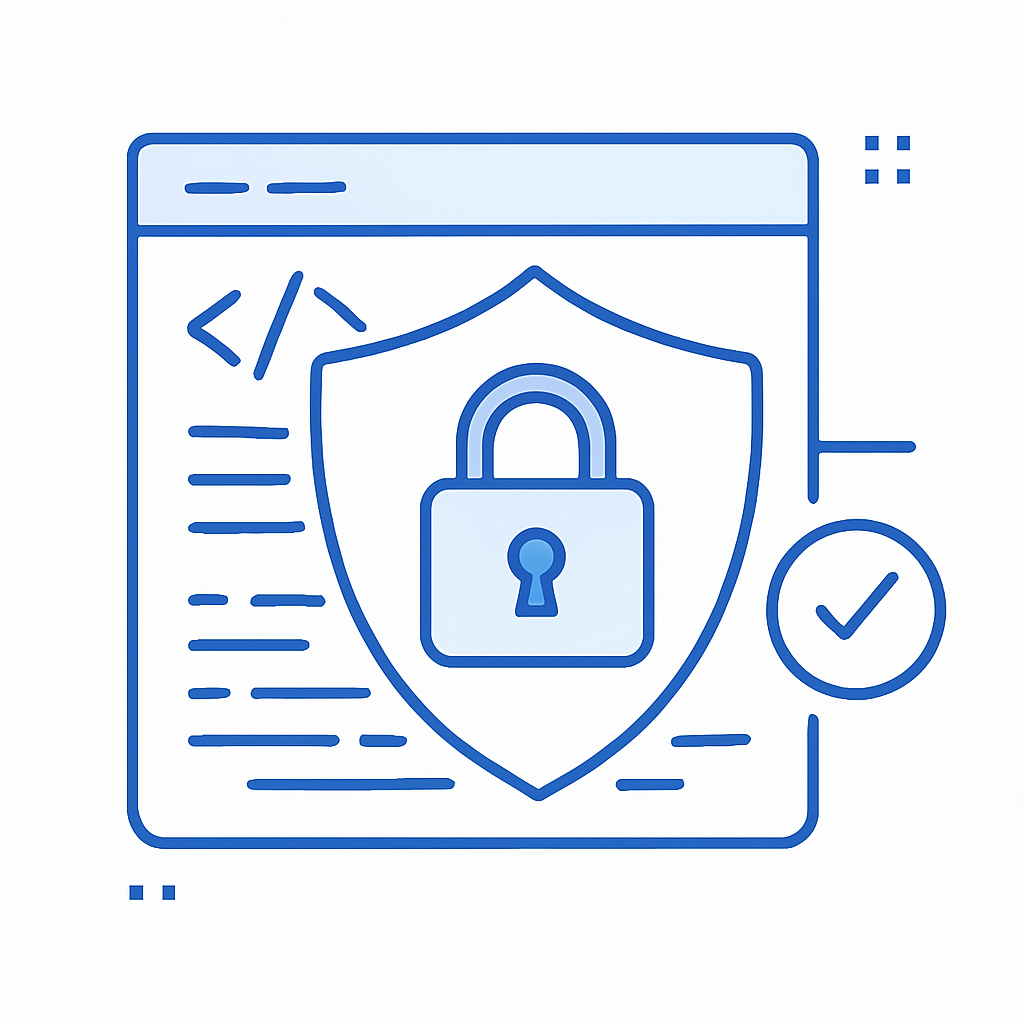
I. Introduction: Defining Secure Coding Practices
Secure coding refers to the practice of writing source code for software applications in a manner that actively prevents the introduction of security vulnerabilities. It is a proactive approach integrated throughout the software development lifecycle (SDLC), aiming to build applications resilient against malicious attacks and safeguarding the confidentiality, integrity, and availability (CIA) of data and system resources. This involves adhering to established guidelines and best practices designed to minimize security risks from the initial design phase through implementation, testing, and deployment.
The core objective of secure coding is to prevent common software weaknesses that can be exploited by attackers. These weaknesses often arise from coding errors, design flaws, or misconfigurations. By focusing on security at every stage, developers can build software that inherently protects against unauthorized access, data breaches, denial-of-service attacks, and other cyber threats. This contrasts sharply with traditional development approaches where security might be treated as an afterthought, addressed only late in the cycle or after deployment.
B. Critical Importance in Modern Software Development
In today's interconnected digital landscape, the importance of secure coding cannot be overstated. Software applications underpin critical infrastructure, financial systems, healthcare services, and countless aspects of daily life. Attacks targeting application-layer vulnerabilities are increasingly common and sophisticated, with studies indicating that a significant percentage of internet attack attempts target web applications. Failure to implement secure coding practices leaves applications susceptible to exploitation, potentially leading to severe consequences.
The prevalence of vulnerabilities stems partly from the inherent complexity of modern software and the common practice of using third-party libraries and frameworks, which can introduce their own security risks if not properly vetted and managed. Furthermore, the pressure for rapid development cycles can sometimes lead to security being overlooked. Secure coding provides a necessary discipline to counteract these pressures and build more trustworthy systems.
C. Economic and Reputational Impact of Insecure Code
The consequences of insecure code extend far beyond technical issues. Security breaches resulting from software vulnerabilities can lead to significant financial losses due to remediation costs, regulatory fines (e.g., under GDPR, HIPAA, PCI DSS), legal liabilities, and operational disruption. Studies have shown that fixing security flaws discovered late in the development cycle or after deployment is substantially more expensive—potentially up to 100 times more—than addressing them during the design or coding phases.
Beyond direct financial costs, security incidents severely damage an organization's reputation and erode customer trust. Rebuilding trust after a breach is a challenging and lengthy process. Conversely, demonstrating a commitment to security through rigorous secure coding practices can enhance brand reputation and provide a competitive advantage.
D. The "Shift-Left" Imperative
The recognition of the high cost and impact of fixing vulnerabilities late in the SDLC has led to the "Shift-Left" security movement. This paradigm advocates for integrating security considerations and activities as early as possible in the development process—shifting them leftward on the typical SDLC timeline. Secure coding is a fundamental component of the shift-left approach. By embedding security into requirements gathering, design, and implementation, organizations can proactively prevent vulnerabilities rather than reactively fixing them. This leads to more robust, reliable, and trustworthy software delivered more efficiently and cost-effectively.
II. Fundamental Principles of Secure Coding
Building secure software relies on adhering to a set of fundamental principles that guide development decisions and practices. These principles form the bedrock of secure coding, aiming to minimize the attack surface and mitigate potential vulnerabilities.
A. Input Validation
A foundational principle is the rigorous validation of all input data received by an application. Untrusted input, whether from users, external systems, files, or databases, is a primary vector for attacks like Injection (including SQL Injection and XSS) and buffer overflows.
Effective input validation involves several key techniques:
- Server-Side Enforcement: All critical validation must occur on a trusted system, typically the server-side, as client-side validation can be easily bypassed.
- Allowlisting (Positive Validation): Define precisely what constitutes valid input (e.g., allowed characters, formats, lengths, ranges) and reject anything that does not conform. This is significantly more secure than denylisting (trying to block known bad patterns), which is often incomplete and easily circumvented.
- Canonicalization: Convert input data to a standard, simplified form (e.g., decoding URL or HTML encoding, standardizing character sets like UTF-8) before validation to prevent attackers from bypassing checks using encoding tricks. Validation should occur after decoding.
- Type, Length, and Range Checks: Verify that input data conforms to the expected data type, falls within acceptable length limits, and is within a valid range or set of values.
- Centralized Routine: Implement a centralized validation routine or library to ensure consistency across the application.
- Handling Specific Characters: Explicitly check for potentially harmful characters like null bytes, newline characters, and path traversal sequences (../) if they cannot be disallowed by the allowlist.
All validation failures should result in the rejection of the input, and these failures should be logged securely.
B. Output Encoding
Complementary to input validation, output encoding ensures that data sent from the application, particularly data that originated from untrusted sources, is treated as data and not as executable code by the recipient (typically a user's browser). This is the primary defense against Cross-Site Scripting (XSS) attacks.
Key aspects of output encoding include:
- Contextual Encoding: The encoding method must be appropriate for the context in which the data will be rendered (e.g., HTML body, HTML attributes, JavaScript, CSS, URL parameters). Using the wrong encoding type can be ineffective or even introduce new vulnerabilities. HTML entity encoding is common but not universally sufficient.
- Server-Side Execution: Encoding operations must be performed on a trusted system (the server) immediately before the data is sent to the client.
- Use Standard Libraries: Rely on standard, well-tested encoding libraries (e.g., OWASP Java Encoder, libraries built into modern web frameworks) rather than attempting custom encoding routines.
- Encode Untrusted Data: All data originating from untrusted sources that is included in output must be encoded. This includes data retrieved from databases or external systems if its origin or content cannot be fully trusted.
- Sanitization for Specific Interpreters: For data destined for interpreters like SQL, LDAP, or OS command shells, contextual sanitization (escaping specific metacharacters) is necessary in addition to input validation.
C. Authentication and Password Management
Authentication is the process of verifying the claimed identity of a user, service, or system. Secure applications require robust authentication mechanisms to prevent unauthorized access.
Best practices include:
- Strong Authentication Mechanisms: Implement secure protocols, such as multi-factor authentication (MFA), wherever possible, especially for sensitive accounts or operations.
- Secure Password Storage: Never store passwords in plaintext or using reversible encryption. Use strong, adaptive, one-way hashing algorithms with a unique salt per user (e.g., Argon2id, bcrypt, scrypt, PBKDF2). Hashing must be performed server-side.
- Password Policies: Enforce policies regarding password length (minimum and maximum), discouraging easily guessable passwords by checking against breach lists, and allowing a wide range of characters. Avoid arbitrary complexity rules and mandatory periodic changes unless compromise is suspected.
- Secure Credential Handling: Transmit credentials only over encrypted channels (TLS/HTTPS) using POST requests. Protect against brute-force and credential stuffing attacks using account lockout, throttling, and CAPTCHAs.
- Secure Recovery: Implement secure mechanisms for password reset/recovery, using temporary, time-limited, single-use tokens sent to verified channels.
- Centralized Implementation: Use a centralized, standard, well-tested authentication service or library across the application. Authentication controls must be enforced server-side.
D. Authorization and Access Control
Authorization determines what actions an authenticated user is permitted to perform and what resources they can access. It works in conjunction with authentication to enforce security policies.
Fundamental access control practices include:
- Server-Side Enforcement: Authorization decisions must be made and enforced on the server-side using trusted information (e.g., server-side session data). Client-side checks are insufficient.
- Enforce on Every Request: Authorization checks must be performed on every request for a protected resource or function, as attackers can bypass intended workflows.
- Principle of Least Privilege: Grant users and system components only the minimum permissions necessary to perform their required tasks.
- Role-Based Access Control (RBAC): Implement RBAC or similar models to manage permissions based on user roles rather than individual users, simplifying administration and reducing errors.
- Deny by Default: Access should be denied unless explicitly granted ("fail-secure").
- Centralized Component: Use a single, site-wide component or library for performing authorization checks.
- Protect Access Control Mechanisms: Restrict access to the policy information, configuration, and attributes used to make authorization decisions.
- Secure Direct Object References: Prevent users from accessing resources they are not authorized for by simply changing an identifier (e.g., in a URL or form parameter). Implement checks to verify the user's permission for the specific object being requested.
E. Session Management
Session management involves securely handling the lifecycle of a user's authenticated session after login. Weak session management can lead to session hijacking or fixation attacks.
Secure practices entail:
- Strong Session Identifiers: Generate session IDs on a trusted system (server) using a cryptographically secure random number generator. IDs should be long and unpredictable.
- Secure Transmission and Storage: Transmit session IDs only over encrypted channels (TLS/HTTPS) and store them securely, typically in HTTP cookies with appropriate attributes (Secure, HttpOnly, SameSite). Do not expose session IDs in URLs, logs, or error messages.
- Session Timeouts: Implement reasonably short inactivity timeouts and enforce absolute session timeouts to limit the window for hijacking.
- Session ID Regeneration: Generate a new session ID upon successful login (to prevent session fixation) and potentially periodically during the session or upon privilege changes.
- Secure Logout: Ensure logout functionality fully invalidates the session on the server-side.
- Concurrency Control: Consider disallowing concurrent logins with the same user ID.
- CSRF Protection: Supplement session management with anti-CSRF tokens for state-changing requests.
F. Error Handling and Logging
Secure error handling prevents the leakage of sensitive information that could aid attackers, while robust logging provides audit trails for detecting and investigating security incidents.
Key guidelines include:
- Minimal Information Disclosure: Error messages shown to users should be generic and not reveal internal system details, stack traces, database errors, or sensitive data. Use custom error pages.
- Secure Defaults: Error handling logic, especially for security controls, should default to denying access.
- Centralized Logging: Implement a central routine for logging operations.
- Log Sufficient Detail: Log critical security events (both success and failure), including authentication attempts, access control decisions, input validation failures, exceptions, and administrative actions. Logs should include timestamps, user IDs, source IPs, event descriptions, and severity levels.
- Protect Log Data: Do not log sensitive information like passwords, session IDs, or excessive system details. Restrict access to log files to authorized personnel only. Ensure log viewing tools are secure against injection (e.g., XSS via log data). Consider log integrity mechanisms.
- Resource Management: Ensure resources like memory are properly freed during error conditions.
G. Principle of Least Privilege
This fundamental principle dictates that any user, program, or process should only have the minimum level of access (privileges) necessary to perform its intended function. Adhering to this principle limits the potential damage if a component or user account is compromised. It applies broadly, including database access permissions, file system access, API access rights, and user roles within the application. Privileges should be elevated only when necessary and dropped as soon as possible.
H. Defense in Depth
This principle advocates for layering multiple, independent security controls to protect system resources. The idea is that if one security layer fails or is bypassed, other layers are still in place to prevent or impede an attack. Secure coding practices contribute to application-level defenses (e.g., input validation, output encoding, access control), which should complement network-level security (firewalls), platform hardening, and operational security measures.
III. Common Vulnerabilities Addressed by Secure Coding (OWASP Top 10 Focus)
Secure coding practices are specifically designed to prevent or mitigate common software vulnerabilities that attackers frequently exploit. The OWASP Top 10 list provides a widely recognized benchmark of the most critical security risks facing web applications, based on broad consensus and data analysis. Understanding these vulnerabilities is crucial for prioritizing secure coding efforts.
A. Overview of OWASP Top 10 (2021)
The OWASP Top 10 is updated periodically to reflect the evolving threat landscape. The 2021 version introduced significant changes, including new categories and shifts in ranking, emphasizing issues like insecure design and software integrity alongside implementation flaws. The 2021 list includes:
- A01: Broken Access Control
- A02: Cryptographic Failures
- A03: Injection
- A04: Insecure Design
- A05: Security Misconfiguration
- A06: Vulnerable and Outdated Components
- A07: Identification and Authentication Failures
- A08: Software and Data Integrity Failures
- A09: Security Logging and Monitoring Failures
- A10: Server-Side Request Forgery (SSRF)
B. Detailed Analysis of Key Vulnerabilities
A01:2021 - Broken Access Control
This category moved to the top spot in 2021 due to its high prevalence (found in 94% of applications tested). It occurs when restrictions on what authenticated users are allowed to do are not properly enforced. Attackers can exploit these flaws to access unauthorized functionality or data, such as accessing other users' accounts, viewing sensitive files, modifying other users' data, or changing access rights. Examples include modifying URL parameters or API requests to access resources without proper checks, privilege escalation, or Insecure Direct Object References (IDOR). Mitigation relies heavily on server-side enforcement of authorization rules based on user roles and privileges, adhering to the principle of least privilege, denying access by default, and using mechanisms like Role-Based Access Control (RBAC). Verifying authorization on every request using trusted session data is critical. Tools like Infrastructure as Code (IaC) scanners and penetration testing can help identify these flaws.
A02:2021 - Cryptographic Failures
Previously named "Sensitive Data Exposure," this category focuses on failures related to cryptography itself or its absence, often leading to data exposure. It involves issues like transmitting data in cleartext (especially sensitive data like credentials or PII), storing data without proper encryption, using weak or outdated cryptographic algorithms (e.g., MD5, SHA1 for hashing; DES, RC4 for encryption), poor key management practices, or using default/hardcoded keys. Mitigation involves encrypting sensitive data both at rest and in transit using strong, current algorithms (e.g., AES-256, TLS 1.2+) and protocols (HTTPS, HSTS), employing robust key management practices (secure generation, storage, rotation, destruction), using strong salted password hashing (Argon2id, bcrypt), and avoiding unnecessary storage of sensitive data. Disabling caching for sensitive information is also recommended.
A03:2021 - Injection
Injection flaws occur when untrusted input is processed by an interpreter as part of a command or query, leading to unintended execution. This category remains highly prevalent (94% tested) and now explicitly includes Cross-Site Scripting (XSS) alongside classic injections like SQL, NoSQL, OS Command, and LDAP injection. Attackers can exploit these to steal data, modify data, gain unauthorized access, or execute arbitrary code. Mitigation requires a combination of server-side input validation/sanitization, using safe APIs that avoid direct interpretation of untrusted data (like parameterized queries/prepared statements for SQL injection), contextual output encoding (especially for XSS), and applying the principle of least privilege to limit the impact of a successful injection. Modern frameworks often provide built-in protections.
A04:2021 - Insecure Design
This new category highlights vulnerabilities stemming from fundamental flaws in the software's design and architecture, which cannot be fixed by perfect implementation alone. It emphasizes the need to integrate security considerations early in the SDLC ("shift left"). Examples include insecure business logic flows, inadequate threat modeling leading to missing security controls, reliance on insecure mechanisms like weak password recovery questions, or overly complex architectures that expand the attack surface. Mitigation requires proactive measures like systematic threat modeling during the design phase, applying secure design principles (e.g., defense in depth, least privilege, secure defaults), utilizing secure design patterns and reference architectures, and conducting thorough security architecture reviews.
A05:2021 - Security Misconfiguration
This risk involves improperly configured security controls or insecure default settings across the application stack, including the OS, frameworks, libraries, databases, web servers, and cloud services. It is highly prevalent (90% tested) and includes the former XML External Entities (XXE) category. Examples include leaving default credentials unchanged, enabling unnecessary services or features, overly permissive access controls (e.g., on cloud storage), missing security patches, verbose error messages revealing internal details, or insecure configurations in frameworks or servers. Mitigation involves establishing secure baseline configurations, implementing repeatable hardening processes (ideally automated), disabling unused features/accounts, regularly patching and updating all components, performing configuration reviews, using automated tools to scan for misconfigurations (e.g., IaC scanners), and setting appropriate security headers.
A06:2021 - Vulnerable and Outdated Components
This category addresses the risk of using software components (libraries, frameworks, OS components, etc.) with known vulnerabilities. Given the heavy reliance on third-party and open-source software in modern development, this is a significant attack vector. Attackers actively scan for applications using components with known CVEs. Mitigation requires maintaining an accurate inventory of all components and their versions (e.g., a Software Bill of Materials - SBoM), regularly scanning for vulnerabilities using Software Composition Analysis (SCA) tools, promptly updating or patching vulnerable components, removing unused dependencies, and obtaining components only from trusted sources. Pinning dependencies can prevent unexpected updates.
A07:2021 - Identification and Authentication Failures
Formerly "Broken Authentication," this category encompasses weaknesses in identifying users and managing authentication and sessions. Failures can allow attackers to impersonate legitimate users or bypass authentication entirely. Examples include allowing weak passwords, failing to protect against automated attacks like credential stuffing or brute force, improper session invalidation, predictable session tokens, or not implementing MFA. Mitigation involves implementing strong authentication (including MFA), enforcing robust password policies (length, checking against breaches), using secure password storage, implementing secure session management practices (random IDs, timeouts, secure flags), and protecting against automated attacks through rate limiting and account lockout. Using standardized, well-vetted authentication frameworks can help.
A08:2021 - Software and Data Integrity Failures
A new category focusing on failures to protect against violations of software and data integrity. This relates to making assumptions about the integrity of software updates, critical data, and CI/CD pipelines without proper verification. It includes the risk of insecure deserialization, where processing untrusted serialized data can lead to remote code execution or other attacks. This category reflects the growing concern over supply chain attacks. Mitigation involves verifying the integrity of software updates and components using digital signatures or hashes, securing the CI/CD pipeline, using trusted sources for dependencies, implementing secure deserialization practices (validation, safe libraries, class allowlisting), and monitoring for unauthorized changes.
A09:2021 - Security Logging and Monitoring Failures
Expanded from the 2017 list, this highlights the importance of sufficient logging and monitoring to detect attacks, respond to incidents, and perform forensic analysis. Failures include not logging critical events (logins, failures, access violations), logs lacking detail, inadequate monitoring or alerting, insecure log storage, or logs being overwritten too quickly. Mitigation requires logging relevant security events (successes and failures) with adequate context, ensuring logs are protected from tampering and unauthorized access, implementing centralized monitoring and alerting systems, and testing the effectiveness of these systems.
A10:2021 - Server-Side Request Forgery (SSRF)
SSRF vulnerabilities occur when a web application fetches a remote resource based on user-supplied input (like a URL) without proper validation, allowing an attacker to coerce the application into sending crafted requests to arbitrary destinations. This can be used to scan internal networks, access internal services (like metadata services in cloud environments), or interact with other backend systems. Mitigation involves strict server-side validation and sanitization of user-supplied URLs, using explicit allowlists for permitted protocols, domains, and ports, network segmentation to isolate the functionality, enforcing deny-by-default firewall rules, and avoiding sending raw server responses back to the client.
C. Interrelation between Principles and Vulnerabilities
A critical observation is the direct mapping between the fundamental secure coding principles (Section II) and the common vulnerabilities outlined in the OWASP Top 10. Failures in applying these principles often manifest as specific, exploitable vulnerabilities. For instance:
- Inadequate Input Validation and Output Encoding directly lead to Injection flaws (A03), including SQLi and XSS.
- Violations of Authentication, Authorization, Session Management, and Least Privilege principles result in Broken Access Control (A01) and Identification and Authentication Failures (A07).
- Incorrect application of Cryptographic Practices manifests as Cryptographic Failures (A02).
- Poor Error Handling and Logging contributes to Security Logging and Monitoring Failures (A09) and can exacerbate other vulnerabilities by leaking information.
- Ignoring Least Privilege or secure defaults contributes to Security Misconfiguration (A05).
This strong correlation underscores that mastering and consistently applying the fundamental principles is paramount to mitigating the most prevalent and critical web application security risks. The introduction of categories like Insecure Design (A04) and Software and Data Integrity Failures (A08) further highlights that security must be considered beyond just implementation-level coding, encompassing architecture, threat modeling, and the entire software supply chain. Addressing these requires a holistic approach involving secure design practices, rigorous verification of components and updates, and securing the development and deployment pipelines themselves. Mitigation, therefore, necessitates a multi-layered strategy combining secure coding techniques, secure configuration management, appropriate tooling (like SCA, SAST, DAST), and robust development processes.
IV. Secure Coding Techniques in Practice
Translating secure coding principles into practice requires specific techniques tailored to different aspects of application development.
A. Robust User Input Handling
Building upon the principle of input validation, robust handling involves applying specific strategies:
- Leverage Framework Features: Utilize the built-in validation capabilities provided by development frameworks (e.g., Django Validators, Apache Commons Validators, Jakarta Bean Validation), as these are often well-tested and maintained.
- Careful Use of Regular Expressions: For validating structured data, use precisely crafted regular expressions. Ensure patterns are anchored (using ^ and $) to match the entire string and avoid overly permissive wildcards (like .). Be mindful of potential Regular Expression Denial of Service (ReDoS) vulnerabilities with complex or inefficient patterns.
- Secure File Uploads: Implement multiple checks for file uploads: validate file types using content inspection (magic numbers/MIME types) rather than relying solely on extensions, enforce strict size limits, scan uploads for malware, store files using server-generated, non-user-controlled filenames, and restrict execute permissions on upload directories. If handling archives like ZIP files, validate contents (paths, compression ratio, size) before extraction. For images, use rewriting libraries to validate and sanitize content.
- Email Address Validation: Combine basic syntactic checks (presence of '@', reasonable length, allowed characters in domain) with a verification process involving sending a unique, time-limited, single-use token or link to the provided address to confirm ownership.
- API Input Validation: Treat all data received through API endpoints (parameters, headers, request bodies) as untrusted. Apply rigorous validation and sanitization routines, potentially using schema validation (e.g., JSON Schema).
B. Secure Password and Credential Management
Effective credential security goes beyond basic hashing and involves managing the entire lifecycle of passwords and other secrets:
Secure Storage:
- Hashing: Employ strong, adaptive, salted, one-way hash functions like Argon2id (preferred), scrypt, bcrypt, or PBKDF2. Avoid plaintext, reversible encryption, or weak hashes (MD5, SHA1).
- Salting: Use a unique, random salt for each password, stored alongside the hash.
- Peppering (Optional): Consider a system-wide secret pepper, stored separately from the database, for added defense.
- Work Factors: Configure appropriate iteration counts or cost factors to make hashing computationally expensive for attackers.
Password Policies:
- Length: Enforce a reasonable minimum length (e.g., >= 8, ideally >= 15) and allow for long passphrases (e.g., >= 64).
- Complexity: Avoid mandating specific character types (uppercase, number, symbol). Allow a broad character set (including spaces).
- Rotation: Avoid forced periodic changes; rotate only upon suspected compromise. Prevent immediate reuse.
- Blacklisting: Check against dictionaries of breached passwords and context-specific terms.
Secure Handling:
- Transmission: Use TLS/HTTPS for all credential transmission.
- Protection Against Automation: Implement account lockout, throttling, CAPTCHAs, or risk-based authentication.
- Recovery: Utilize secure reset mechanisms with time-limited, single-use tokens.
- Other Secrets (API Keys, Connection Strings): Do not hardcode secrets. Store them securely using environment variables, encrypted configuration files, or dedicated secrets management systems (e.g., HashiCorp Vault, Azure Key Vault, AWS Secrets Manager). Rotate secrets regularly and use dynamic or temporary credentials where feasible.
C. Securing Database Interactions
Protecting database interactions is critical to prevent data breaches and maintain data integrity.
Preventing SQL Injection:
- Parameterized Queries (Prepared Statements): This is the most effective and recommended technique. Database APIs provide mechanisms to define the SQL query structure separately from the user-supplied data, ensuring the data is treated as literal values and not executable code.
- Object-Relational Mappers (ORMs): ORMs (e.g., Hibernate, Entity Framework, SQLAlchemy) often generate parameterized queries by default, providing significant protection. However, care must be taken when using raw SQL execution features within ORMs.
- Stored Procedures: Can be secure if implemented correctly (i.e., without constructing dynamic SQL inside the procedure using user input). They centralize database logic.
- Input Validation as Defense-in-Depth: While parameterization is primary, validating input intended for queries provides an additional layer of defense.
Applying Least Privilege:
Configure database connection accounts with the minimum permissions required for the application's functionality. Use separate accounts for different levels of access (e.g., read-only vs. read-write).
Secure Database Configuration:
Use strong, unique credentials for database access and store connection strings securely (not hardcoded). Change default administrative passwords, disable unused features and default accounts/schemas.
D. Applying Cryptography Correctly
Using cryptography effectively requires careful selection of algorithms, secure key management, and correct implementation.
Algorithm and Mode Selection:
Use standard, well-vetted, strong algorithms (AES, RSA >= 2048 bits, ECC Curve25519, SHA-2/3, Argon2id, etc.). Avoid deprecated (MD5, SHA1, DES) or custom algorithms. For block ciphers like AES, use secure modes, preferably authenticated encryption modes like GCM or CCM. Use secure random padding (OAEP for RSA). Consult NIST guidelines (FIPS 140-2, SP 800-57).
Key Management Lifecycle:
This is paramount and includes:
- Generation: Use cryptographically secure random number generators (CSPRNGs) within FIPS 140-2 validated modules.
- Storage: Protect keys rigorously. Use Hardware Security Modules (HSMs) or dedicated secrets management systems. Never store keys in plaintext or embed in code. Encrypt stored keys with KEKs of equal or greater strength.
- Distribution: Use secure protocols.
- Rotation: Rotate keys periodically based on risk assessment and policy.
- Destruction: Securely destroy keys when no longer needed.
- Auditing: Log key management events.
Correct Implementation:
Perform crypto operations server-side when protecting secrets from the user. Use unique, random nonces/IVs as required by the cipher mode. Use standard, validated cryptographic libraries.
E. Leveraging Secure Libraries and Framework Features
A recurring theme across these techniques is the importance of utilizing secure, well-established libraries and framework features instead of attempting manual implementations of complex security controls like input validation, password hashing, parameterized queries, or cryptography. Modern frameworks often incorporate security features by default (e.g., ORMs using parameterized queries, template engines providing output encoding). Developers should prioritize understanding and correctly using these built-in features, as they are typically developed and vetted by security experts. However, reliance is not absolute; developers must understand the limitations and potential bypasses, ensuring features are configured securely and not inadvertently disabled.
V. Contextual Secure Coding: Languages and Platforms
While fundamental security principles are universal, their practical application and the specific vulnerabilities encountered vary significantly depending on the programming language, platform, and application type. Secure coding requires understanding these contextual nuances.
A. C/C++: Memory Safety Challenges
C and C++ offer high performance and low-level system access but lack built-in memory safety guarantees due to manual memory management and pointer arithmetic. This makes them susceptible to critical vulnerabilities:
- Buffer Overflows: Writing data beyond the allocated bounds of a buffer (array), potentially overwriting adjacent memory, corrupting data, or executing arbitrary code. This often results from using unsafe functions like strcpy or incorrect index/pointer calculations.
- Use-After-Free/Dangling Pointers: Accessing memory after it has been deallocated (free() or delete), leading to crashes or potential exploitation if an attacker can control the reallocated memory content.
- Memory Leaks: Failing to deallocate memory that is no longer needed, leading to resource exhaustion and potential denial of service.
Mitigation Strategies for C/C++:
- Bounds Checking: Rigorously check array indices and pointer calculations before access.
- Safe Functions: Use safer library functions that incorporate size limits (e.g., strncpy, snprintf, memcpy_s), but understand their limitations and potential pitfalls (e.g., null termination with strncpy).
- Modern C++ Practices: Utilize C++ features like smart pointers (std::unique_ptr, std::shared_ptr) for automatic resource management, standard library containers (std::vector, std::string) instead of raw arrays/pointers, and references where appropriate. Avoid manual new/delete or malloc/free when possible.
- Secure Coding Standards: Adhere to standards like CERT C/C++ or MISRA C/C++, which provide specific rules to avoid memory errors, integer overflows, insecure string handling, etc.
- Tooling: Employ static analysis (SAST) tools specifically designed for C/C++ to detect potential memory safety issues early. Use dynamic analysis tools like AddressSanitizer during testing.
B. Java: Common Pitfalls and Secure Practices
Java benefits from automatic garbage collection, which eliminates many C/C++ style memory management errors like use-after-free. However, resource leaks (e.g., file handles, network connections) can still occur if not managed correctly (e.g., using try-with-resources). Java applications, especially web applications, face other significant risks:
- Injection Vulnerabilities: SQL, Command, LDAP, and JPA Query Language (JPA QL) injection remain threats if dynamic queries are constructed unsafely. Mitigation relies on using PreparedStatement for SQL, safe APIs for OS commands, and proper input validation.
- Insecure Deserialization: Java's native serialization mechanism is notoriously risky when processing untrusted data, potentially leading to remote code execution. Mitigation involves avoiding native serialization with untrusted input, using safer formats like JSON with secure libraries (e.g., Jackson), implementing class allowlisting, or using look-ahead validation.
- Dependency Vulnerabilities: Java ecosystems rely heavily on third-party libraries (JARs). Keeping these dependencies updated and scanned for known vulnerabilities (using tools like OWASP Dependency-Check) is crucial.
- Standard Web Vulnerabilities: XSS, CSRF, Access Control issues require standard mitigations like output encoding (e.g., OWASP Java Encoder), anti-CSRF tokens, and robust authentication/authorization using frameworks like Spring Security.
- Cryptography: Use standard JCA/JCE providers or secure libraries (e.g., Google Tink), avoid weak algorithms/modes (like ECB), manage keys securely, and configure TLS correctly (JSSE).
Adhering to the CERT Oracle Secure Coding Standard for Java provides language-specific guidance.
C. Python: Security Considerations
Python's dynamic typing and extensive libraries offer rapid development but require attention to specific security aspects:
- Injection: While ORMs (SQLAlchemy, Django ORM) provide good protection against SQLi by default, vulnerabilities arise if raw SQL is used improperly or if command injection occurs via unsafe use of os.system or subprocess with external input. Parameterized queries (cursor.execute("...?", (param,))) are essential when not using an ORM. Avoid eval() with untrusted data.
- Insecure Deserialization: The pickle module is unsafe for untrusted data; prefer safer formats like JSON.
- Dependency Management: The Python Package Index (PyPI) hosts numerous packages. Use tools like pip-audit, Safety, or Snyk to scan for vulnerabilities in dependencies and keep them updated.
- Template Security: Use template engines (Jinja2, Django) that provide automatic HTML escaping to prevent XSS. Be wary of Server-Side Template Injection (SSTI) if user input is insecurely embedded in templates.
- Secrets Management: Avoid hardcoding credentials. Use environment variables (loaded via python-dotenv), configuration files with appropriate permissions, or dedicated secrets management tools.
- Randomness: Use the secrets module for cryptographic purposes, not the random module.
Referencing general OWASP guidelines and Python-specific security resources is recommended.
D. JavaScript (Web): Mitigating XSS and CSRF
JavaScript security in the web context is dominated by client-side vulnerabilities, primarily XSS and CSRF, due to its role in manipulating the browser DOM and handling user interactions.
Cross-Site Scripting (XSS):
Occurs when malicious scripts are injected into a website and executed by a victim's browser.
Prevention: Requires contextual output encoding (HTML entity encoding, JavaScript escaping, etc.) for all user-controlled data rendered on the page. Use modern frameworks (React, Angular, Vue) that often provide automatic encoding. Implement a strong Content Security Policy (CSP) via HTTP headers to restrict script sources and execution. Avoid dangerous JavaScript functions/properties like innerHTML, document.write, and eval() with untrusted input; use safer alternatives like textContent or createElement. Sanitize HTML input using libraries like DOMPurify, especially for DOM-based XSS, but prioritize server-side validation and output encoding.
Cross-Site Request Forgery (CSRF):
Tricks an authenticated user's browser into sending an unwanted request to a web application.
Prevention: The primary defense is the Synchronizer Token Pattern: embed a unique, unpredictable, secret token in forms/requests for state-changing actions and verify it server-side. Use the SameSite cookie attribute (Strict or Lax) to prevent the browser from sending session cookies with cross-origin requests. Checking Origin or Referer headers can be a secondary defense but is less reliable. Require re-authentication for highly sensitive operations.
E. Mobile Platforms (Android/iOS): Key Security Controls (OWASP MASVS)
Mobile applications present unique challenges due to platform interactions, local data storage, IPC, and binary distribution. The OWASP Mobile Application Security Verification Standard (MASVS) provides a comprehensive framework. Key areas include:
- Secure Data Storage (MASVS-STORAGE): Protecting sensitive data stored locally using platform-specific secure storage APIs (e.g., Android Keystore, iOS Keychain) and appropriate encryption.
- Cryptography (MASVS-CRYPTO): Correctly implementing standard cryptographic algorithms and secure key management.
- Authentication & Session Management (MASVS-AUTH): Securely handling user credentials, managing sessions, and utilizing platform authentication features (e.g., biometrics) appropriately.
- Network Communication (MASVS-NETWORK): Ensuring all network traffic uses TLS, implementing certificate pinning where appropriate, and validating server certificates.
- Platform Interaction (MASVS-PLATFORM): Securely using platform APIs, managing permissions, securing Inter-Process Communication (IPC), and handling WebViews safely.
- Code Quality (MASVS-CODE): Applying general secure coding practices like input validation, managing dependencies securely, and implementing mechanisms for secure updates.
- Resilience (MASVS-RESILIENCE): Implementing measures like obfuscation, anti-debugging, and integrity checks to hinder reverse engineering and tampering (though fixing underlying vulnerabilities is generally prioritized).
F. Embedded Systems: Addressing Constraints (MISRA, CERT C)
Embedded systems often operate under tight resource constraints (memory, power, CPU) and may have stringent safety and reliability requirements (e.g., automotive, medical). C and C++ are prevalent, making memory safety a primary concern.
- MISRA C/C++: Focuses on code safety, reliability, and portability by restricting ambiguous or potentially unsafe language features (e.g., pointer arithmetic, dynamic memory allocation, recursion). Widely used in automotive and other safety-critical domains.
- CERT C/C++: Focuses specifically on security vulnerabilities in C/C++, providing rules and recommendations against common errors like buffer overflows, integer issues, and insecure function usage. It has a broader security scope than MISRA.
- Practices: Development in these environments often mandates adherence to MISRA or CERT standards, enforced through rigorous static analysis. Careful resource management, secure update mechanisms, and minimizing the attack surface are crucial.
The choice of language and platform significantly influences the specific vulnerabilities that are most likely and the most effective mitigation techniques. While C/C++ demands meticulous attention to memory management, Java introduces risks like deserialization. Web development with JavaScript necessitates strong defenses against XSS and CSRF. Mobile and embedded systems require adherence to platform-specific guidelines and standards like MASVS, MISRA, or CERT C. Modern languages and frameworks often provide security advantages (like garbage collection or built-in ORMs) but do not eliminate the need for secure coding practices; developers must understand how to use these features correctly and be aware of potential bypasses or misconfigurations.
VI. Adhering to Secure Coding Standards and Guidelines
To ensure consistency, effectiveness, and compliance, development teams should adhere to established secure coding standards and guidelines. These standards provide structured frameworks, codify best practices, and serve as benchmarks for code reviews and testing.
A. The Landscape of Standards
Several organizations publish widely recognized standards and guidelines:
- OWASP (Open Web Application Security Project): A leading authority, particularly for web and mobile security. Key resources include the OWASP Top 10, ASVS, MASVS/MASTG, Secure Coding Practices Checklist, Cheat Sheet Series, and SAMM.
- CERT/CC (SEI, Carnegie Mellon): Publishes language-specific secure coding standards (C, C++, Java, Perl, Android) focusing on preventing common programming errors that lead to vulnerabilities.
- NIST (National Institute of Standards and Technology): Provides crucial standards and guidelines for cryptography, key management, password security (SP 800-63b), and secure development frameworks (SSDF).
- MISRA (Motor Industry Software Reliability Association): Defines coding guidelines for C/C++ primarily aimed at safety, reliability, and portability in embedded systems, particularly automotive.
- CWE (Common Weakness Enumeration): Provides a standardized dictionary of software weaknesses, often used as a reference by other standards and tools (e.g., CWE/SANS Top 25).
- Industry/Regulatory Standards: Requirements like PCI DSS (Payment Card Industry), HIPAA (Health Insurance Portability and Accountability Act), and GDPR (General Data Protection Regulation) often mandate secure development practices.
B. Key Standards Deep Dive
OWASP Secure Coding Practices Checklist:
Overview: This guide provides a technology-agnostic, checklist-formatted set of general secure coding practices designed for easy integration into the SDLC. It focuses on requirements rather than specific exploits. While the original project is archived, its content has been migrated into the broader OWASP Developer Guide. Downloads of the v2.1 PDF are still referenced.
Key Areas Covered: The checklist is organized into sections covering fundamental security areas: Input Validation, Output Encoding, Authentication and Password Management, Session Management, Access Control, Cryptographic Practices, Error Handling and Logging, Data Protection, Communication Security, System Configuration, Database Security, File Management, Memory Management (relevant for languages like C/C++), and General Coding Practices. Each section contains specific, actionable checklist items.
CERT Secure Coding Standards:
Focus: These standards provide detailed, language-specific rules and recommendations for C, C++, Java, Perl, and Android to prevent common programming errors that lead to security vulnerabilities and undefined behaviors. They are developed through a community process led by the Software Engineering Institute (SEI) at Carnegie Mellon University.
Structure: The standards consist of "Rules" (violations likely cause defects, conformance checkable via inspection) and "Recommendations" (conformance improves security, violation not necessarily a defect).
Risk Assessment: Each rule includes a risk assessment based on Severity, Likelihood, and Remediation Cost, resulting in a priority level (1-3) to help teams focus efforts on the most critical issues.
Availability: The standards are available online via the CERT Secure Coding wiki, with older versions sometimes available as PDF downloads.
C. Utilizing Standards Effectively
Standards should not be applied blindly. Organizations should select standards relevant to their technology stack, application type, and risk profile. Often, a combination of standards provides the best coverage (e.g., OWASP Top 10 for awareness, ASVS for requirements, CERT C for C implementation details). Standards should be integrated into developer training, code review checklists, and automated testing tool configurations. They provide objective criteria for evaluating code security.
VII. Verification and Enforcement Mechanisms
Adhering to secure coding principles and standards requires verification and enforcement throughout the SDLC. Various tools and methodologies are employed to detect vulnerabilities and ensure compliance.
A. Static Application Security Testing (SAST)
SAST tools, also known as static code analyzers or white-box testing tools, examine application source code, bytecode, or binaries without executing the application. They build a model of the code and data flow and apply predefined rules to identify patterns indicative of potential security vulnerabilities.
Strengths:
- Early Detection: Can be integrated early in the SDLC, often directly into developer IDEs or CI/CD pipelines, providing immediate feedback. This makes remediation cheaper and faster.
- Code-Level Identification: Pinpoints the exact location (file, line number) of potential flaws, aiding developer remediation.
- Broad Code Coverage: Analyzes the entire codebase, potentially finding vulnerabilities in less-frequently executed paths.
- Common Vulnerability Detection: Effective at finding certain types of vulnerabilities like buffer overflows, SQL injection patterns, and use of insecure functions.
Weaknesses:
- False Positives: Prone to reporting issues that are not actual exploitable vulnerabilities, requiring significant effort to triage results.
- Runtime Blindness: Cannot detect vulnerabilities that only manifest at runtime, such as authentication flaws, access control issues, configuration errors, or vulnerabilities arising from interactions between components.
- Language Dependency: SAST tools are typically specific to certain programming languages.
- Build/Compilation Requirements: Many tools require code that can be compiled or built, which can be a challenge.
Example Tools: SonarQube, Checkmarx, Veracode Static Analysis, OpenText Fortify SCA, Semgrep, Gosec, Coverity, and many others.
B. Dynamic Application Security Testing (DAST)
DAST tools, also known as black-box testing tools, interact with a running application from the outside, simulating attacks against its exposed interfaces (web pages, APIs) without knowledge of the internal source code. They send malicious or unexpected inputs and analyze the application's responses to identify vulnerabilities.
Strengths:
- Runtime Vulnerability Detection: Effective at finding issues that only appear during execution, such as configuration errors, authentication/session management problems, and vulnerabilities resulting from interactions between different components or systems.
- Language Agnostic: Since they test the running application, DAST tools are independent of the underlying programming language or framework.
- Lower False Positive Rate (Generally): Issues identified by DAST often represent exploitable vulnerabilities, leading to fewer false positives compared to SAST.
- Real-World Attack Simulation: Tests the application as an attacker would see it.
Weaknesses:
- Late SDLC Integration: Requires a running application, so typically used later in the SDLC (testing, staging, production), making fixes more costly. Efforts exist to "shift DAST left".
- No Code Visibility: Cannot pinpoint the exact location of the vulnerability within the source code, making remediation more difficult for developers.
- Limited Code Coverage: Only tests exposed interfaces and functionalities exercised during the scan; may miss vulnerabilities in unexercised code paths.
- Potential for Disruption: Active scanning can potentially impact application performance or stability, often requiring testing in dedicated environments.
Example Tools: OWASP ZAP (Zed Attack Proxy), Burp Suite, Acunetix, Veracode Dynamic Analysis, HCL AppScan, Fortify WebInspect, Nuclei.
C. Interactive Application Security Testing (IAST)
IAST combines elements of SAST and DAST by using instrumentation (agents or sensors) deployed within the running application during testing (e.g., QA, functional testing). These agents monitor code execution, data flow, and interactions in real-time, identifying vulnerabilities based on actual runtime behavior.
Strengths:
- Accuracy (Low False Positives): By analyzing runtime behavior and code context simultaneously, IAST significantly reduces false positives compared to SAST.
- Code-Level Remediation Guidance: Like SAST, IAST can often pinpoint the vulnerable line of code.
- Runtime Context: Detects vulnerabilities that depend on runtime conditions and data flow, similar to DAST.
- Real-Time Feedback: Provides results quickly during the testing phase, integrating well with CI/CD pipelines and DevOps workflows.
- Broad Vulnerability Coverage: Can detect a wide range of issues, including those in custom code and libraries.
Weaknesses:
- Performance Overhead: Instrumentation agents can introduce some performance overhead on the application during testing.
- Language Dependency: Requires agents compatible with the application's language and framework.
- Test Coverage Dependency: Effectiveness relies on the thoroughness of the functional tests executed while the IAST agent is active. Vulnerabilities in unexercised code paths may be missed.
- Setup Effort: Requires deploying and configuring agents within the application environment.
Example Tools: Checkmarx CxIAST, Synopsys Seeker IAST, Contrast Assess, Datadog Application Vulnerability Management.
D. Secure Code Reviews (Manual and Hybrid)
Process: Involves human reviewers examining source code to identify security flaws, logic errors, and deviations from secure coding standards. This can be purely manual or hybrid (human review augmented by automated tool findings).
Importance: Essential for finding complex vulnerabilities, business logic flaws, and subtle errors that automated tools often miss. Provides context and understanding of "real risk". Crucial for validating fixes and ensuring adherence to standards.
Best Practices:
- Prioritization: Focus review efforts on high-risk code segments (authentication, authorization, input handling, cryptography, data access).
- Checklists & Standards: Use checklists based on standards like OWASP (Top 10, ASVS, Secure Coding Practices) or internal guidelines to ensure consistency and coverage.
- Training: Ensure reviewers are trained in secure coding practices and common vulnerabilities.
- Automation Assist: Use SAST/SCA tools to handle common checks, allowing human reviewers to focus on complex issues and tool findings validation.
- Integration: Embed code reviews into the development workflow (e.g., pull requests).
- Documentation: Document findings clearly, including vulnerability details, impact, and remediation recommendations.
OWASP Resource: The OWASP Code Review Guide provides detailed methodology and guidance.
E. Complementary Nature of Verification Methods
No single testing method finds all vulnerabilities. SAST excels at early code-level checks, DAST finds runtime and configuration issues, IAST provides runtime context with code visibility, and manual reviews catch logic flaws and complex errors. A comprehensive application security program utilizes a combination of these approaches (often alongside Software Composition Analysis - SCA for dependencies) integrated throughout the SDLC to provide layered defense and maximize vulnerability detection.
VIII. Integrating Secure Coding into the Software Development Lifecycle (SDLC)
Secure coding is most effective when it is not an isolated activity but an integral part of the entire Software Development Lifecycle (SDLC). Integrating security practices throughout the SDLC, often referred to as a Secure SDLC (SSDLC), helps identify and mitigate risks early, reducing costs and improving the overall security posture.
A. Secure SDLC Models Overview
Several established models provide frameworks for integrating security into the SDLC:
- Microsoft Security Development Lifecycle (SDL): A pioneering and widely adopted prescriptive model introduced in 2004. It defines specific security practices and requirements for each phase of development, emphasizing training, threat modeling, secure coding standards, security testing, and incident response. While comprehensive, its structured nature might be perceived as less flexible for highly agile DevOps environments.
- OWASP Software Assurance Maturity Model (SAMM): A flexible, measurable framework designed to help organizations assess and improve their software security posture over time. It organizes security practices into business functions (Governance, Design, Implementation, Verification, Operations) with defined maturity levels, allowing organizations to tailor their improvement roadmap. Its adaptability aligns well with DevOps principles.
- Building Security In Maturity Model (BSIMM): A descriptive model based on observing the actual software security practices of numerous organizations. It catalogs common activities, helping organizations compare their practices against industry norms and identify potential areas for improvement.
These models provide valuable structures, but the key is embedding security thinking and activities consistently throughout the development process.
B. Integration Points Across Phases
Security activities should be woven into each phase of a standard SDLC:
- Training: Foundational and continuous training for developers, testers, and architects on secure coding principles, common vulnerabilities (e.g., OWASP Top 10), threat modeling, secure design, and the use of security tools is essential.