Comprehensive Guide to API Error Code Management
Mastering API error codes is essential for building robust and user-friendly applications. This comprehensive guide explores best practices for handling and documenting errors, ensuring clear communication between your API and its users.
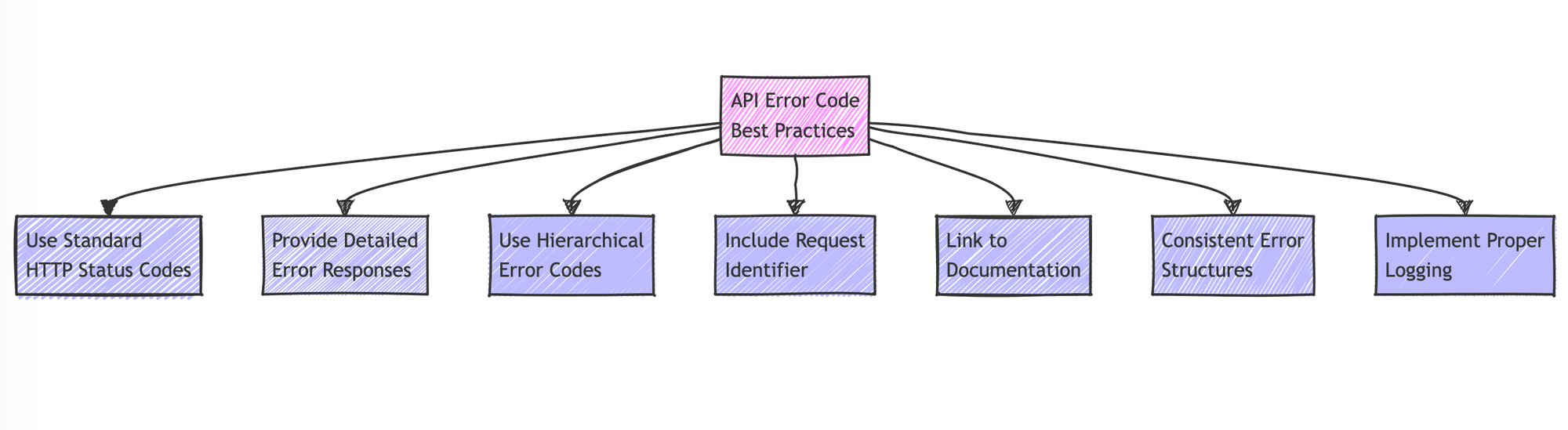
Error handling is a critical aspect of API design and development. Well-designed error codes and messages can significantly improve the developer experience, reduce support overhead, and enhance the overall quality of your API. This guide will walk you through the best practices for creating and managing error codes in a developer-oriented API system.
Best Practices for API Error Codes
a. Use Standard HTTP Status Codes
Always use standard HTTP status codes as the first line of error reporting. These are widely understood and provide a broad categorization of the error.
HTTP/1.1 404 Not Found
b. Provide Detailed Error Responses
Include a detailed error response in the body of your HTTP response. This should be a structured object (typically JSON) containing more specific information about the error.
{
"error": {
"code": "RESOURCE_NOT_FOUND",
"message": "The requested resource could not be found.",
"details": "User with ID 12345 does not exist in the system.",
"timestamp": "2023-08-09T14:30:00Z",
"request_id": "f7a8b9c0-d1e2-3f4g-5h6i-7j8k9l0m1n2o"
}
}
c. Use Hierarchical Error Codes
Implement a hierarchical error code system. This allows for both broad and specific error categorization.
Example:
- AUTH_ERROR
- AUTH_INVALID_CREDENTIALS
- AUTH_EXPIRED_TOKEN
- AUTH_INSUFFICIENT_PERMISSIONS
d. Include a Request Identifier
A Request Identifier, often called a Request ID, is a unique string or number assigned to each API request. Always include a unique identifier for each request. Its primary purpose is to provide a way to track and correlate requests across systems, which is incredibly useful for debugging, logging, and monitoring.
Example of Request Identifier in an API response:
{
"data": {
"user_id": 12345,
"username": "johndoe"
},
"meta": {
"request_id": "550e8400-e29b-41d4-a716-446655440000"
}
}
Examples of Request Identifiers:
Hierarchical identifier (for microservices):
gateway-123:auth-456:user-789
Combination of service name and random number:
API-789456123
Base64-encoded random string:
dGhpc2lzYW5leGFtcGxl
Timestamp-based identifier:
20230809-154322-789
This combines a date (20230809), time (154322), and a random number (789).
UUID (Universally Unique Identifier):
550e8400-e29b-41d4-a716-446655440000
This is a common format due to its uniqueness and standardization.
e. Provide Links to Documentation
Include links to relevant documentation in your error responses. This can help developers quickly find information on how to resolve the error.
{
"error": {
"code": "RATE_LIMIT_EXCEEDED",
"message": "You have exceeded your rate limit.",
"documentation_url": "https://api.example.com/docs/errors/rate-limiting"
}
}
f. Use Consistent Error Structures
Maintain a consistent structure for all error responses across your API. This predictability helps developers in handling errors programmatically.
g. Implement Proper Logging
Ensure comprehensive logging on the server-side. While the client receives a sanitized error message, log detailed error information server-side for debugging and monitoring.
Things to Avoid
a. Exposing Sensitive Information
Never include sensitive information like stack traces, server paths, or database queries in error responses.
b. Using Ambiguous Error Codes
Avoid generic error codes like "ERROR_001". These provide no context and make debugging difficult.
c. Inconsistent Naming Conventions
Don't mix naming conventions. Stick to one style (e.g., UPPER_SNAKE_CASE) for all error codes.
d. Changing Error Codes Frequently
Changing error codes can break client integrations. Avoid changing existing error codes unless absolutely necessary.
Making It Developer-Friendly
a. Provide Clear and Actionable Error Messages
Error messages should clearly state what went wrong and, if possible, how to fix it.
{
"error": {
"code": "INVALID_PARAMETER",
"message": "The 'email' parameter is invalid.",
"details": "Please provide a valid email address in the format user@example.com."
}
}
b. Offer Multiple Languages
Consider providing error messages in multiple languages. Use content negotiation to determine the appropriate language.
c. Implement Retry-After Headers
For rate limiting or temporary server issues, include a Retry-After
header to indicate when the client should retry the request.
HTTP/1.1 429 Too Many Requests
Retry-After: 30
d. Provide SDK Support
Develop SDKs for popular programming languages that handle error parsing and provide language-specific exceptions.
Future-Proofing Your Error Codes
a. Use Versioning
Implement versioning in your API, including error responses. This allows you to evolve your error handling without breaking existing integrations.
b. Design for Extensibility
Structure your error responses to allow for easy addition of new fields in the future.
{
"error": {
"code": "PAYMENT_FAILED",
"message": "The payment could not be processed.",
"details": {
"reason": "Insufficient funds",
"transaction_id": "1234567890"
},
"additional_info": {} // Placeholder for future extensions
}
}
c. Implement Feature Flags
Use feature flags to gradually roll out changes to error handling, allowing for easy rollback if issues arise.
Examples from Industry Leaders
Stripe
Stripe's API is renowned for its developer-friendly error handling:
- They use standard HTTP status codes.
- Error types are clearly categorized (e.g.,
card_error
,validation_error
). - Detailed error messages and suggestions are provided.
- They include a unique error ID for tracking.
Example Stripe error:
{
"error": {
"code": "resource_missing",
"doc_url": "https://stripe.com/docs/error-codes/resource-missing",
"message": "No such customer: cus_12345",
"param": "customer",
"type": "invalid_request_error"
}
}
b. GitHub
GitHub's API error responses are clear and actionable:
- They use a consistent error object structure.
- Error messages are human-readable and often suggest a solution.
- They provide links to relevant documentation.
Example GitHub error:
{
"message": "Validation Failed",
"errors": [
{
"resource": "Issue",
"field": "title",
"code": "missing_field"
}
],
"documentation_url": "https://docs.github.com/rest/reference/issues#create-an-issue"
}
Implementing Error Codes
a. Define an Error Code Enum
Create an enumeration of all possible error codes. This ensures consistency and makes it easier to manage codes.
from enum import Enum
class ErrorCode(Enum):
RESOURCE_NOT_FOUND = "RESOURCE_NOT_FOUND"
INVALID_REQUEST = "INVALID_REQUEST"
RATE_LIMIT_EXCEEDED = "RATE_LIMIT_EXCEEDED"
INTERNAL_SERVER_ERROR = "INTERNAL_SERVER_ERROR"
# ... more error codes ...
b. Create an Error Response Class
Implement a class to generate consistent error responses:
from dataclasses import dataclass
from typing import Optional, Any
import time
import uuid
@dataclass
class ErrorResponse:
code: ErrorCode
message: str
details: Optional[str] = None
timestamp: float = time.time()
request_id: str = str(uuid.uuid4())
additional_info: dict = field(default_factory=dict)
def to_dict(self) -> dict:
return {
"error": {
"code": self.code.value,
"message": self.message,
"details": self.details,
"timestamp": self.timestamp,
"request_id": self.request_id,
"additional_info": self.additional_info
}
}
c. Implement Error Handling in Your API
Use the ErrorResponse
class in your API endpoints:
from fastapi import FastAPI, HTTPException
from fastapi.responses import JSONResponse
app = FastAPI()
@app.exception_handler(HTTPException)
async def http_exception_handler(request, exc):
error_response = ErrorResponse(
code=ErrorCode.RESOURCE_NOT_FOUND if exc.status_code == 404 else ErrorCode.INTERNAL_SERVER_ERROR,
message=str(exc.detail),
details=f"An error occurred while processing the request: {exc.detail}"
)
return JSONResponse(status_code=exc.status_code, content=error_response.to_dict())
@app.get("/users/{user_id}")
async def get_user(user_id: int):
# Simulating a user not found scenario
if user_id == 0:
raise HTTPException(status_code=404, detail="User not found")
# ... rest of the function ...
This setup ensures that all errors are consistently formatted and contain the necessary information for debugging and client-side error handling.
Conclusion
Implementing a robust error handling system is crucial for creating a developer-friendly API. By following these best practices, avoiding common pitfalls, and learning from industry leaders, you can create an API that is not only powerful but also a joy for developers to work with. Remember, good error handling is an ongoing process – continuously gather feedback from your API consumers and iterate on your error reporting to provide the best possible developer experience.