Authentication and Single Sign-On: Essential Technical Foundations
Dive deep into the technical fundamentals of Authentication and SSO systems. Learn how HTTP, security protocols, and best practices work together to create robust authentication solutions for modern web applications.
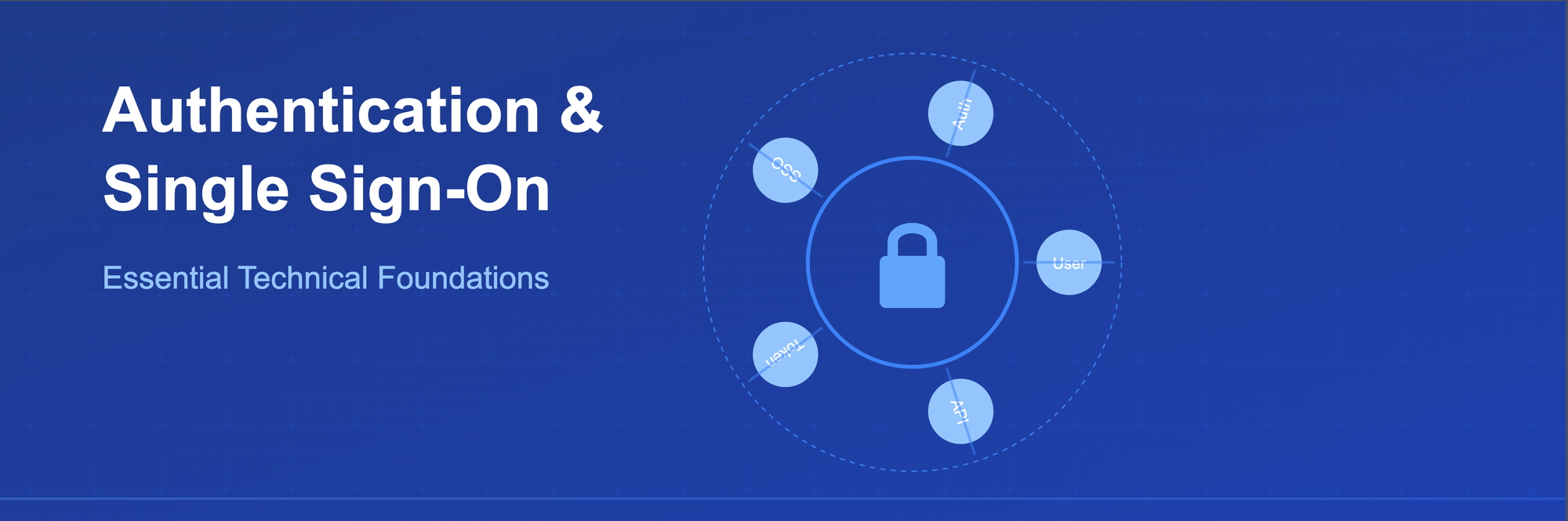
Authentication and Single Sign-On (SSO) are fundamental aspects of modern web security, but implementing them effectively requires a deep understanding of various web technologies and security concepts. This guide explores the essential technical foundations that every developer should master before working with authentication systems.
Essentials to know before implementing Auth and SSO
- Understanding HTTP
- Cookies and Session Management
- Database Security
- Authentication Security: Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- Cross-Origin Resource Sharing (CORS)
- Single Sign-On Tokens
- Best Practices and Security Checklist
1. Understanding HTTP - The Foundation of Web Authentication
Authentication in web applications doesn't exist in isolation - it's built upon the fundamental protocol that powers the web: HTTP. Before we can understand how authentication works, we need to grasp how HTTP shapes our approach to security and user identification.
HTTP serves as the backbone of web communication, but its stateless nature presents unique challenges for authentication. Imagine trying to maintain a conversation where the other person forgets everything after each sentence - that's essentially what we're dealing with in HTTP. This characteristic has profound implications for how we design authentication systems.
Think of HTTP as the postal service of the internet. Just as a mail carrier delivers letters without knowing their content or context, HTTP delivers requests and responses without inherently maintaining any memory of previous interactions. This is why we need additional mechanisms to maintain user sessions and authentication states.
HTTP Protocol Basics
The Hypertext Transfer Protocol (HTTP) forms the backbone of data communication on the web. To understand authentication, we must first grasp how HTTP works:
- Stateless Nature: HTTP is inherently stateless, meaning each request is independent and carries no information about previous requests. This characteristic presents unique challenges for authentication, which requires maintaining user state across requests.
- Request-Response Cycle: Every HTTP interaction consists of:
- A request from the client containing:
- Method (GET, POST, etc.)
- Headers
- URL
- Optional body
- A response from the server containing:
- Status code
- Headers
- Body
- A request from the client containing:
- Headers in Authentication:
Authorization
: Carries credentials for authenticationCookie
: Maintains session stateOrigin
: Important for CORS securityContent-Type
: Specifies the format of request/response data
HTTP Authentication Schemes
HTTP provides several built-in authentication schemes:
- Simple but sends credentials with every request
- Base64 encoding is not encryption
- Should only be used over HTTPS
- Common in OAuth 2.0 and JWT implementations
- Token-based approach
- More secure than Basic Authentication
- Digest Authentication:
- Uses cryptographic hashing
- More secure than Basic Authentication
- Less common in modern applications
Bearer Authentication:
Authorization: Bearer <token>
Basic Authentication:
Authorization: Basic base64(username:password)
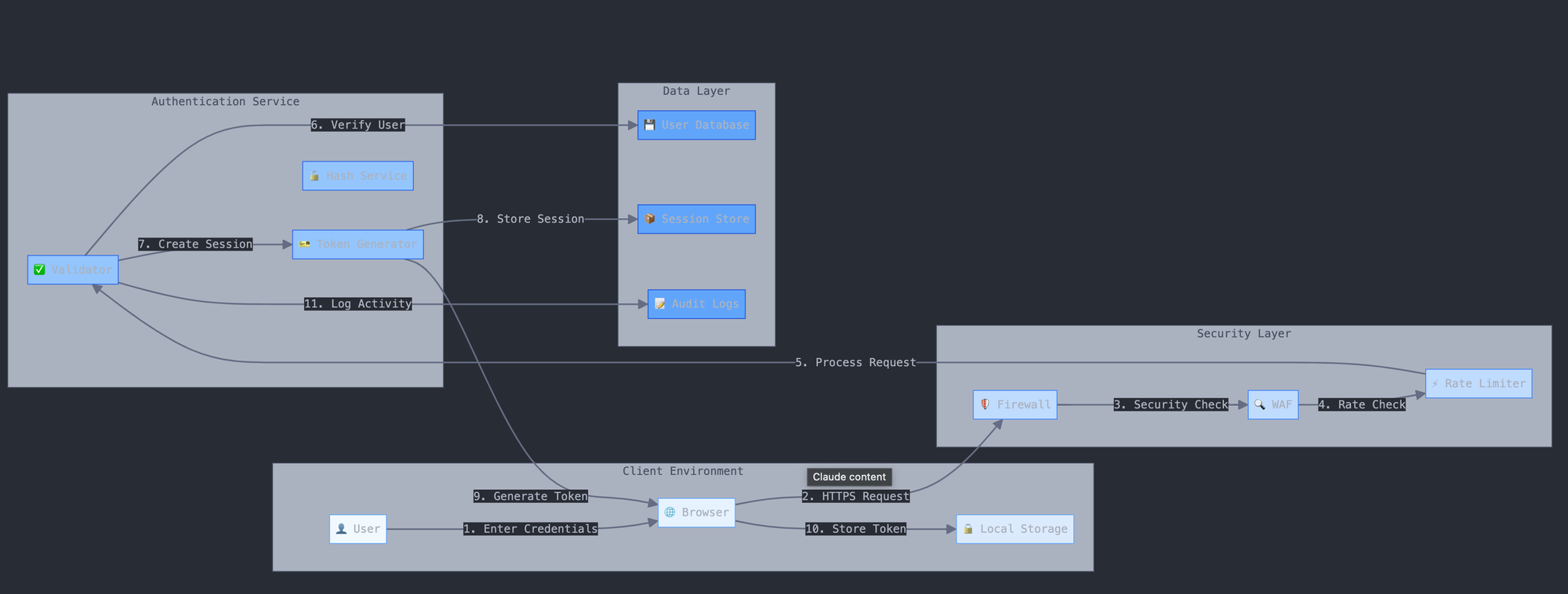
2. Cookies and Session Management
Cookies and session management form the backbone of maintaining user authentication states in web applications. Despite HTTP's stateless nature, cookies provide the crucial ability to remember who a user is between requests, making them fundamental to modern authentication systems.
Understanding cookies in authentication is like understanding how a hotel key card system works. The key card (cookie) proves you're a guest, tracks which room is yours (session data), and has built-in security features (cookie attributes). Just as a hotel carefully manages its key card system, web applications must carefully manage their cookies and sessions.
Session management builds upon cookies to create a complete system of user identity tracking. Think of it as the difference between simply having a key card and having a complete system that knows which key cards are active, when they were issued, and when they should expire. This comprehensive management is crucial for maintaining security while providing a seamless user experience.
Cookie Security
Cookies are crucial for session management in authentication systems. Here's how to use them securely:
Session Cookie vs. Persistent Cookie:
// Session cookie (expires when browser closes)
Set-Cookie: sessionId=abc123; HttpOnly; Secure
// Persistent cookie (explicit expiry)
Set-Cookie: rememberMe=true; Max-Age=2592000; HttpOnly; Secure
Secure Cookie Configuration:
// Express.js example
app.use(session({
cookie: {
secure: true, // Only transmit over HTTPS
httpOnly: true, // Prevent JavaScript access
sameSite: 'strict', // Protect against CSRF
maxAge: 3600000, // 1 hour expiry
domain: '.example.com', // Scope to domain
path: '/' // Accessible across all paths
}
}));
Session Management Best Practices
Session Storage:
// Redis example
const Redis = require('ioredis');
const redis = new Redis();
async function storeSession(sessionId, userData) {
await redis.setex(`session:${sessionId}`, 3600, JSON.stringify(userData));
}
Session ID Generation:
const crypto = require('crypto');
function generateSessionId() {
return crypto.randomBytes(32).toString('hex');
}
3. Database Security
SQL Injection attacks target the very foundation of user authentication - the database where user credentials and permissions are stored. This type of attack is particularly insidious because it exploits the trust between your application and its database.
In the context of authentication, SQL injection can be catastrophic because it potentially gives attackers access to user credentials, session tokens, and permission levels. It's like having a master key that not only opens any door but can also reprogram all the locks in the building.
The importance of preventing SQL injection in authentication systems cannot be overstated. Your authentication system might have perfect session management and robust password hashing, but if an attacker can directly manipulate your database queries, these protections become meaningless. It's similar to having a sophisticated alarm system but leaving the database of security codes written on a public whiteboard.
SQL Injection Fundamentals
SQL injection attacks can compromise authentication systems by manipulating database queries:
-- Vulnerable query
SELECT * FROM users WHERE username = '$username' AND password = '$password'
-- Attack input
username: admin' --
password: anything
-- Resulting query
SELECT * FROM users WHERE username = 'admin' -- ' AND password = 'anything'
Prevention Techniques
Input Validation:
function validateUsername(username) {
// Only allow alphanumeric and underscore
return /^[a-zA-Z0-9_]+$/.test(username);
}
ORM Usage:
// Using an ORM like Sequelize
const user = await User.findOne({
where: {
username: username,
passwordHash: hash
}
});
Prepared Statements:
// Node.js example with prepared statements
const query = 'SELECT * FROM users WHERE username = ? AND password_hash = ?';
connection.query(query, [username, passwordHash], function(error, results) {
if (error) throw error;
// Handle results safely
});
4. Authentication Security: Cross-Site Scripting (XSS)
Cross-Site Scripting represents one of the most critical security vulnerabilities in web authentication systems. Think of XSS as an uninvited guest at a party who convinces other guests they're the host - it's a breach of trust that can have severe consequences for security.
In authentication contexts, XSS is particularly dangerous because it can compromise the very tokens and credentials that prove a user's identity. Imagine having a secure vault (your authentication system) but leaving copies of the key (authentication tokens) where attackers can easily find them - that's what happens when XSS vulnerabilities exist in your application.
The relationship between XSS and authentication security is crucial because authentication mechanisms often rely on storing and transmitting sensitive data in the browser. When an XSS vulnerability exists, it's like having a secure door but leaving the windows wide open - attackers can bypass all your careful authentication measures.
Understanding XSS
Cross-Site Scripting attacks occur when malicious scripts are injected into trusted websites. In authentication contexts, XSS can be particularly dangerous:
Types of XSS:a) Reflected XSS:
// Vulnerable code
document.write('<input value="' + userInput + '">');
// Safe code
element.textContent = userInput;
b) Stored XSS:
// Vulnerable pattern
database.store(userInput);
page.innerHTML = database.getData();
// Safe pattern
database.store(sanitizeHTML(userInput));
page.textContent = database.getData();
c) DOM-based XSS:
// Vulnerable
element.innerHTML = window.location.hash.substring(1);
// Safe
element.textContent = window.location.hash.substring(1);
XSS Prevention in Authentication
Input Sanitization:
function sanitizeInput(input) {
return input.replace(/[&<>"']/g, function(match) {
const escape = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": '''
};
return escape[match];
});
}
Content Security Policy (CSP):
Content-Security-Policy: default-src 'self';
script-src 'self' trusted.com;
style-src 'self';
Token Storage:
// Never store sensitive tokens in localStorage
localStorage.setItem('authToken', token); // Vulnerable to XSS
// Instead use httpOnly cookies
// Set-Cookie: authToken=xyz; httpOnly; secure; sameSite=strict
5. Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery represents a subtle but dangerous threat to authentication systems. Unlike other attacks that try to steal credentials, CSRF tricks authenticated users into performing actions they didn't intend. It's like a malicious actor using someone else's already-signed paperwork to authorize transactions they never approved.
In authentication systems, CSRF is particularly concerning because it exploits the trust between the user's browser and your application. When users are authenticated, their browsers automatically include authentication cookies with every request. CSRF attacks take advantage of this automatic inclusion to perform unauthorized actions while the user is authenticated.
The challenge with CSRF in authentication systems is maintaining the balance between user convenience and security. We want users to stay authenticated for a reasonable time, but this same persistence creates opportunities for CSRF attacks. It's similar to wanting a door to automatically unlock for authorized personnel while ensuring no one can trick the system into unlocking at the wrong time.
CSRF Vulnerability
CSRF attacks trick authenticated users into performing unwanted actions:
<!-- Malicious form on attacker's site -->
<form action="https://bank.com/transfer" method="POST">
<input type="hidden" name="amount" value="1000">
<input type="hidden" name="to" value="attacker">
</form>
<script>document.forms[0].submit();</script>
CSRF Prevention
Double Submit Cookie Pattern:
// Set CSRF token in cookie and form
app.get('/form', (req, res) => {
const csrfToken = generateCSRFToken();
res.cookie('XSRF-TOKEN', csrfToken, {
httpOnly: true,
secure: true
});
res.render('form', { csrfToken });
});
Token-based Protection:
// Generate CSRF token
function generateCSRFToken() {
return crypto.randomBytes(32).toString('hex');
}
// Validate token middleware
function validateCSRF(req, res, next) {
if (req.csrfToken() !== req.body._csrf) {
return res.status(403).send('Invalid CSRF token');
}
next();
}
6. Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing is a crucial security feature that directly impacts how authentication systems can be accessed across different domains. In the modern web, where applications often consist of separate frontend and backend services, understanding CORS is essential for building secure authentication systems.
CORS acts like a border control system for web resources. Just as countries have specific rules about who can cross their borders and what they can bring, CORS defines rules about which domains can access your authentication endpoints and what kinds of requests they can make. This is particularly important in authentication systems where you need to carefully control who can send and receive sensitive credentials.
The relationship between CORS and authentication is complex because it affects how your authentication tokens can be transmitted and used. Modern single-page applications and microservices architectures often require careful CORS configuration to ensure that authentication works securely across different domains while preventing unauthorized access.
CORS in Authentication
CORS is crucial for secure cross-origin requests in authentication systems:
Handling Preflight Requests:
app.options('/api/auth', cors()); // Handle preflight for specific route
app.post('/api/auth', cors(), (req, res) => {
// Authentication logic
});
Basic CORS Configuration:
// Express.js CORS setup
app.use(cors({
origin: 'https://trusted-client.com',
methods: ['GET', 'POST'],
allowedHeaders: ['Content-Type', 'Authorization'],
credentials: true
}));
Security Considerations
Credential Handling:
// Client-side fetch with credentials
fetch('https://api.example.com/auth', {
credentials: 'include',
headers: {
'Authorization': `Bearer ${token}`
}
});
Whitelist Approach:
const whitelist = ['https://app1.example.com', 'https://app2.example.com'];
app.use(cors({
origin: function(origin, callback) {
if (whitelist.indexOf(origin) !== -1 || !origin) {
callback(null, true);
} else {
callback(new Error('Not allowed by CORS'));
}
}
}));
7: Single Sign-On Tokens
Single Sign-On represents the evolution of authentication systems from isolated, application-specific solutions to unified, enterprise-wide identity management. SSO is like having a single master key that works across multiple buildings in a campus, rather than carrying different keys for each door.
The value of SSO in modern authentication systems cannot be overstated. It solves several critical problems: reducing password fatigue for users, centralizing authentication control for security teams, and streamlining access management across multiple applications. Think of it as creating a secure, central checkpoint that, once cleared, grants acess to multiple secure areas.
Understanding SSO implementation requires bringing together all the previous concepts - HTTP, cookies, CORS, and security protections - into a cohesive system. It's like orchestrating a complex dance where multiple participants (applications, identity providers, and user browsers) must move in perfect synchronization while maintaining security at every step.
SSO Architecture
OAuth 2.0 Flow:
// OAuth 2.0 Authorization Code Flow
app.get('/auth', (req, res) => {
const authURL = `${idpBaseURL}/oauth/authorize?
client_id=${clientId}&
redirect_uri=${encodeURIComponent(redirectUri)}&
response_type=code&
scope=openid profile email`;
res.redirect(authURL);
});
Identity Provider (IdP) Integration:
// SAML Authentication Example
const saml = require('saml2-js');
const sp_options = {
entity_id: "https://sp.example.com",
private_key: fs.readFileSync("key.pem").toString(),
certificate: fs.readFileSync("cert.pem").toString(),
assert_endpoint: "https://sp.example.com/assert"
};
const sp = new saml.ServiceProvider(sp_options);
Token Management
JWT Handling:
// JWT creation
function createJWT(user) {
return jwt.sign(
{
sub: user.id,
email: user.email,
roles: user.roles
},
process.env.JWT_SECRET,
{
expiresIn: '1h',
audience: 'https://api.example.com'
}
);
}
// JWT verification
function verifyJWT(token) {
try {
return jwt.verify(token, process.env.JWT_SECRET, {
audience: 'https://api.example.com'
});
} catch (err) {
throw new AuthenticationError('Invalid token');
}
}
8. Best Practices and Security Checklist
Security in authentication systems isn't a single feature or setting - it's a comprehensive approach that encompasses all aspects of your application. Best practices serve as your roadmap to creating and maintaining secure authentication systems, helping you avoid common pitfalls and implement proven solutions.
Think of authentication security as a chain where each link represents a different aspect of your system. Just as a chain is only as strong as its weakest link, your authentication system is only as secure as its most vulnerable component. This is why having a comprehensive security checklist and following best practices is crucial.
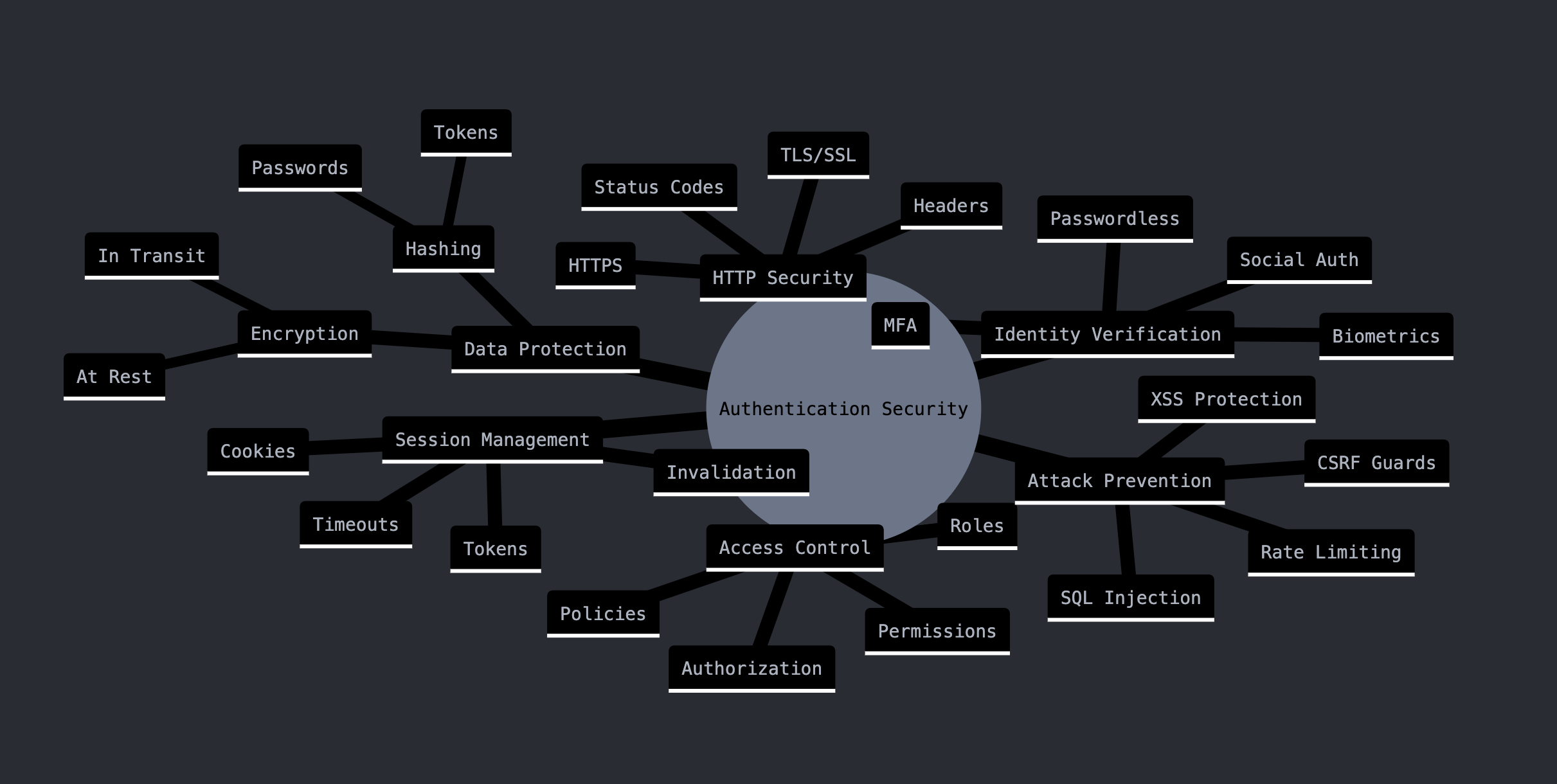
The value of following best practices in authentication cannot be overstated. While it might be tempting to implement quick solutions or take shortcuts, the cost of a security breach far outweighs the time and effort required to implement proper security measures. It's like building a house - taking the time to build on a solid foundation using proven techniques will save you from costly problems in the future.
Authentication Security Checklist
- Password Security:
- Implement strong password policies
- Use secure password hashing (bcrypt/Argon2)
- Enforce MFA for sensitive operations
- Session Security:
- Use secure session storage
- Implement proper session expiration
- Protect against session fixation
- API Security:
- Use rate limiting
- Implement proper error handling
- Log security events
Start Auth NOW
Understanding these fundamental concepts is crucial for implementing secure authentication and SSO systems. Remember to:
- Always use HTTPS
- Implement proper input validation
- Use secure session management
- Keep dependencies updated
- Regularly audit security measures
- Follow security best practices
- Stay informed about new vulnerabilities and attacks
This guide serves as a foundation for building secure authentication systems. As the security landscape evolves, continue to stay updated with the latest security practices and vulnerabilities.