All About Authentication and Authorization Tokens: A Comprehensive Guide
Understand different token types, from bearer tokens to PATs, along with their implementation strategies and security considerations. Perfect for developers, architects, and security professionals building secure systems.
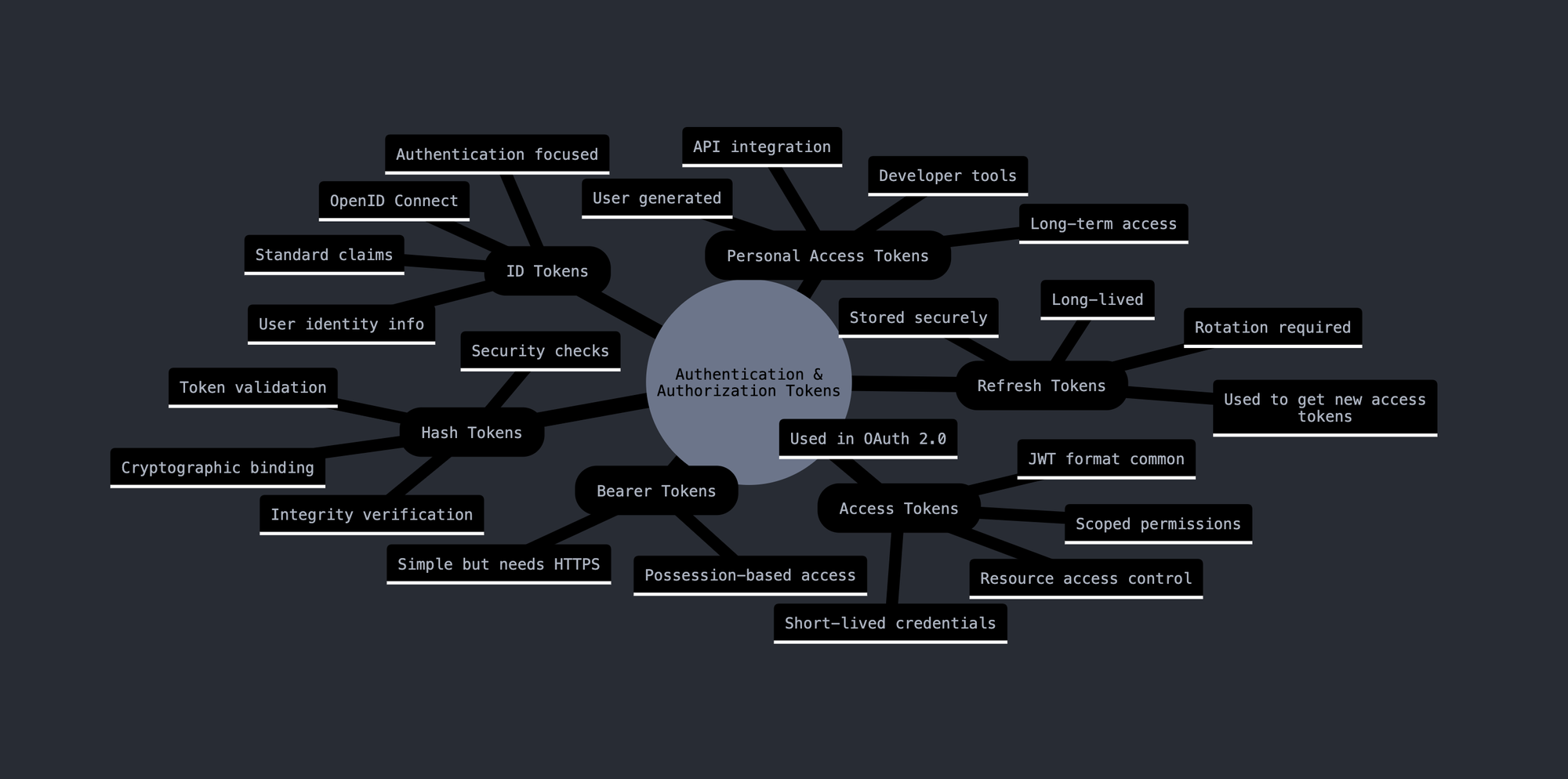
Secure authentication and authorization mechanisms are fundamental to protecting sensitive data and resources. Token-based authentication has emerged as a cornerstone of secure system design, offering a flexible and robust approach to managing user sessions and access rights. This comprehensive guide delves into the world of authentication and authorization tokens, providing developers, architects, and security professionals with the knowledge needed to implement secure token-based systems.
The evolution of authentication mechanisms from simple password-based systems to sophisticated token-based approaches reflects the growing complexity of digital security requirements. As applications become more distributed and cloud-native architectures more prevalent, the need for secure, stateless, and scalable authentication solutions has never been more critical.
Table of Contents
- Introduction
- Core Concepts in Token-Based Authentication
- Types of Tokens
- Bearer Tokens
- Access Tokens
- Refresh Tokens
- ID Tokens
- Hash Tokens
- Personal Access Tokens (PATs)
- Token Implementation and Architecture
- Security Considerations and Best Practices
- Real-World Applications and Use Cases
- Future of Token-Based Authentication
- Conclusion
Core Concepts in Token-Based Authentication
Before diving into specific token types, it's essential to understand the fundamental principles that underpin token-based authentication:
What is a Token?
A token is a piece of data that serves as a security credential for accessing resources or services. Think of it as a digital key that proves the holder's identity and permissions. Unlike traditional session-based authentication, tokens are typically self-contained and carry all necessary information to validate the user's access rights.
Token Characteristics
Several key characteristics define tokens:
- Statelessness: Tokens contain all necessary information within themselves, reducing the need for server-side storage.
- Portability: Tokens can be easily transmitted across different services and systems.
- Time-Bound: Most tokens have an expiration time to limit their potential for misuse.
- Revocability: While tokens themselves are stateless, systems can implement revocation mechanisms when needed.
Types of Tokens
1. Bearer Tokens
Bearer tokens represent one of the most widely used token types in modern web applications, particularly in OAuth 2.0 implementations.
Definition and Structure
A bearer token is a security token that grants access to protected resources based solely on possession. The name "bearer" implies that anyone who possesses the token can use it to access the associated resources, making secure transmission crucial.
httpCopyAuthorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
Advantages
- Simple to implement and use
- Stateless nature reduces server load
- Wide support across frameworks and libraries
Disadvantages
- Vulnerable to theft if not properly secured
- No inherent mechanism to verify the legitimate holder
- Requires secure transmission channels (HTTPS)
2. Access Tokens
Access tokens serve as the primary credentials for accessing protected resources in modern authentication systems.
Structure and Components
Access tokens often take the form of JWTs (JSON Web Tokens) containing three parts:
- Header (algorithm and token type)
- Payload (claims about the user and permissions)
- Signature (for verification)
Implementation Considerations
javascriptCopy// Example JWT structure
{
"header": {
"alg": "HS256",
"typ": "JWT"
},
"payload": {
"sub": "1234567890",
"name": "John Doe",
"scope": ["read:users", "write:users"],
"exp": 1516239022
},
"signature": "..."
}
Key Comparisons
Feature | Bearer Token | Access Token | Hash Token |
---|---|---|---|
Role | Authorization via possession | General access credential | Integrity validation |
Transmission | text Authorization: Bearer | Header, URL, or body12 | Embedded in ID token14 |
Security | Requires HTTPS58 | Varies by type | Cryptographically hashed14 |
Lifespan | Short-lived24 | Short-lived6 | N/A (validation only) |
Structure | Often JWT312 | JWT or opaque string11 | Hash string14 |
3. Refresh Tokens
Refresh tokens provide a mechanism for obtaining new access tokens without requiring user re-authentication.
Purpose and Lifecycle
- Long-lived compared to access tokens
- Used exclusively to obtain new access tokens
- Typically stored securely server-side
- Subject to strict security controls
Security Considerations
- Storage: Must be stored securely, preferably encrypted
- Transmission: Should only be transmitted over secure channels
- Rotation: Regular rotation reduces risk of compromise
- Monitoring: Implement detection for suspicious usage patterns
4. ID Tokens
ID tokens, primarily used in OpenID Connect, provide authentication information about the user.
Characteristics
- Contains user identity information
- Typically implemented as JWTs
- Used for authentication, not authorization
- Include standardized claims about the user
Standard Claims
javascriptCopy{
"iss": "https://auth.example.com",
"sub": "auth0|123456",
"aud": "client_id",
"exp": 1516239022,
"iat": 1516235422,
"name": "John Doe",
"email": "john@example.com"
}
5. Hash Tokens
Hash tokens serve a specific purpose in token validation and integrity checking.
Use Cases
- Token Validation: Verifying the integrity of other tokens
- Cross-Token Binding: Linking different token types together
- Signature Verification: Supporting cryptographic validation
Implementation Example
javascriptCopy// Creating a hash token from an access token
crypto
const crypto = require('crypto');
function generateHashToken(accessToken) {
return .createHash('sha256')
.update(accessToken)
.digest('base64url');
}
6. Personal Access Tokens (PATs)
PATs provide long-term access for automated tools and scripts.
Characteristics
- User-generated
- Long-lived
- Scoped permissions
- Revocable by users
Token Implementation and Architecture
Token Lifecycle Management
- Generation
- Secure random number generation
- Proper encryption
- Inclusion of necessary claims
- Storage
- Server-side considerations
- Client-side storage options
- Security implications
- Validation
- Signature verification
- Expiration checking
- Scope validation
- Revocation
- Revocation mechanisms
- Blacklisting strategies
- Real-time validation
Security Considerations and Best Practices
Security in token-based authentication requires a comprehensive approach that addresses multiple layers of potential vulnerabilities. Understanding and implementing proper security measures is crucial for maintaining the integrity of your authentication system.
Understanding Token Security Threats
Token Theft and Interception
Token theft represents one of the most significant security risks in token-based authentication systems. Attackers can intercept tokens through various sophisticated methods, each requiring specific preventive measures.
Man-in-the-Middle (MITM) Attacks occur when attackers position themselves between the client and server to intercept token transmissions. These attacks are particularly dangerous because they can be difficult to detect. The primary defense against MITM attacks is implementing proper transport layer security through HTTPS and certificate validation. Organizations should also implement HTTP Strict Transport Security (HSTS) to prevent downgrade attacks that force connections back to HTTP.
Cross-Site Scripting (XSS) Attacks pose another significant threat, where malicious scripts can steal tokens stored in client-side storage. The impact of XSS attacks can be minimized through proper token storage strategies, such as using HTTP-only cookies for refresh tokens and keeping access tokens in memory only. Additionally, implementing Content Security Policy (CSP) headers and proper input validation helps prevent script injection attacks.
Token Replay Attacks
Replay attacks occur when intercepted tokens are reused by attackers. These attacks can be particularly problematic because the tokens being used are technically valid. Prevention strategies include:
- Implementing token nonce values that can only be used once
- Including timestamp validation in token verification
- Maintaining a server-side record of used tokens
- Implementing short token expiration times
Implementing Security Best Practices
Token Lifecycle Management
Proper token lifecycle management is crucial for maintaining security. This involves several key aspects:
Token Generation: Tokens should be generated using cryptographically secure random number generators and should include sufficient entropy to prevent guessing attacks.
Token Rotation: Regular rotation of signing keys helps limit the impact of key compromise. This includes maintaining a key rotation schedule and properly handling the transition period between old and new keys.
Token Revocation: A robust system for revoking tokens should be in place, including the ability to revoke individual tokens, all tokens for a specific user, or all tokens across the system in case of a security breach.
Secure Storage Strategies
Implementing secure token storage varies between client and server environments:
Server-side Storage:
- Refresh tokens should be hashed before storage
- Implement proper database security measures
- Use encryption for sensitive token metadata
- Maintain audit logs of token usage
Client-side Storage:
- Store access tokens in memory when possible
- Use secure HTTP-only cookies for refresh tokens
- Implement secure storage mechanisms for mobile applications
- Avoid storing sensitive token information in local storage or session storage
Real-World Applications and Use Cases
Token-based authentication finds practical application in various real-world scenarios, each with its unique requirements and implementation considerations.
Enterprise Single Sign-On (SSO) Implementation
Enterprise SSO systems demonstrate the power of token-based authentication in managing access across multiple applications. In a typical enterprise SSO setup:
Identity Provider (IdP) Integration:
- The IdP serves as the central authentication authority
- User credentials are validated once at the IdP level
- Token-based assertions are generated for accessing various applications
- Applications trust tokens issued by the IdP
Session Management:
- Tokens facilitate seamless navigation between applications
- Session state is maintained across multiple services
- Token expiration and renewal are handled transparently
- Single logout capabilities are implemented across all applications
Microservices Architecture
Token-based authentication is particularly valuable in microservices architectures, where it helps manage secure communication between services:
Service-to-Service Communication:
- Tokens carry service identity and permissions
- Each service can validate tokens independently
- Token propagation maintains security context
- Fine-grained access control between services
Context Propagation:
- User context is maintained across service boundaries
- Authorization decisions can be made at each service level
- Audit trails can be maintained across the entire system
- Performance impact is minimized through efficient token validation
Mobile Application Authentication
Mobile applications present unique challenges for token-based authentication:
Offline Access:
- Tokens enable offline authentication capabilities
- Refresh tokens are securely stored for extended access
- Token renewal handles intermittent connectivity
- Local token validation reduces server load
Device-Specific Considerations:
- Secure storage utilizes platform-specific capabilities
- Biometric authentication can be integrated with token handling
- Multiple devices can be managed for the same user
- Token revocation handles device loss scenarios
Future of Token-Based Authentication
The landscape of token-based authentication continues to evolve with emerging technologies and security requirements.
Decentralized Identity and Blockchain Integration
Blockchain technology is revolutionizing identity management through decentralized approaches:
Decentralized Identifiers (DIDs):
- Users control their own digital identity
- No central authority required for identity verification
- Cryptographic proof of identity ownership
- Immutable audit trail of identity transactions
Verifiable Credentials:
- Digital equivalents of physical credentials
- Cryptographically verified claims
- Privacy-preserving selective disclosure
- Interoperable across different systems
Zero-Knowledge Proofs in Token Authentication
Zero-knowledge proofs represent a breakthrough in privacy-preserving authentication:
Privacy Benefits:
- Prove identity without revealing sensitive information
- Minimize data exposure in authentication processes
- Support selective attribute disclosure
- Enable anonymous authentication
Implementation Considerations:
- Complex cryptographic computations
- Trade-offs between privacy and performance
- Integration with existing systems
- Standardization efforts
Quantum-Resistant Token Algorithms
As quantum computing advances, token-based authentication must evolve to maintain security:
Quantum Threats:
- Current cryptographic algorithms may become vulnerable
- Need for quantum-resistant signature schemes
- Impact on token generation and validation
- Migration strategies for existing systems
Future-Proofing Strategies:
- Development of post-quantum cryptography
- Hybrid classical-quantum approaches
- Updates to token formats and protocols
- Backward compatibility considerations
Conclusion
Token-based authentication has become an integral part of modern application security architecture. Understanding the various token types, their implementations, and security considerations is crucial for building robust and secure systems. As technology evolves, token-based authentication will continue to adapt, incorporating new security measures and addressing emerging threats.
The key to successful token implementation lies in balancing security requirements with usability and performance considerations. By following best practices and staying informed about emerging trends, developers and security professionals can build systems that effectively protect resources while providing a seamless user experience.
Remember that security is an ongoing process, not a one-time implementation. Regular security assessments, updates to token handling mechanisms, and staying current with industry standards are essential for maintaining a robust token-based authentication system.
Additional Resources
For further reading and implementation guidance:
- OAuth 2.0 Specification (RFC 6749)
- JSON Web Token (JWT) Specification (RFC 7519)
- OpenID Connect Core Specification
- NIST Digital Identity Guidelines