SAML (Security Assertion Markup Language): A Comprehensive Guide
Dive into the world of Security Assertion Markup Language (SAML), from its core concepts to practical implementation. Learn how this powerful standard enables secure authentication and single sign-on across different security domains.
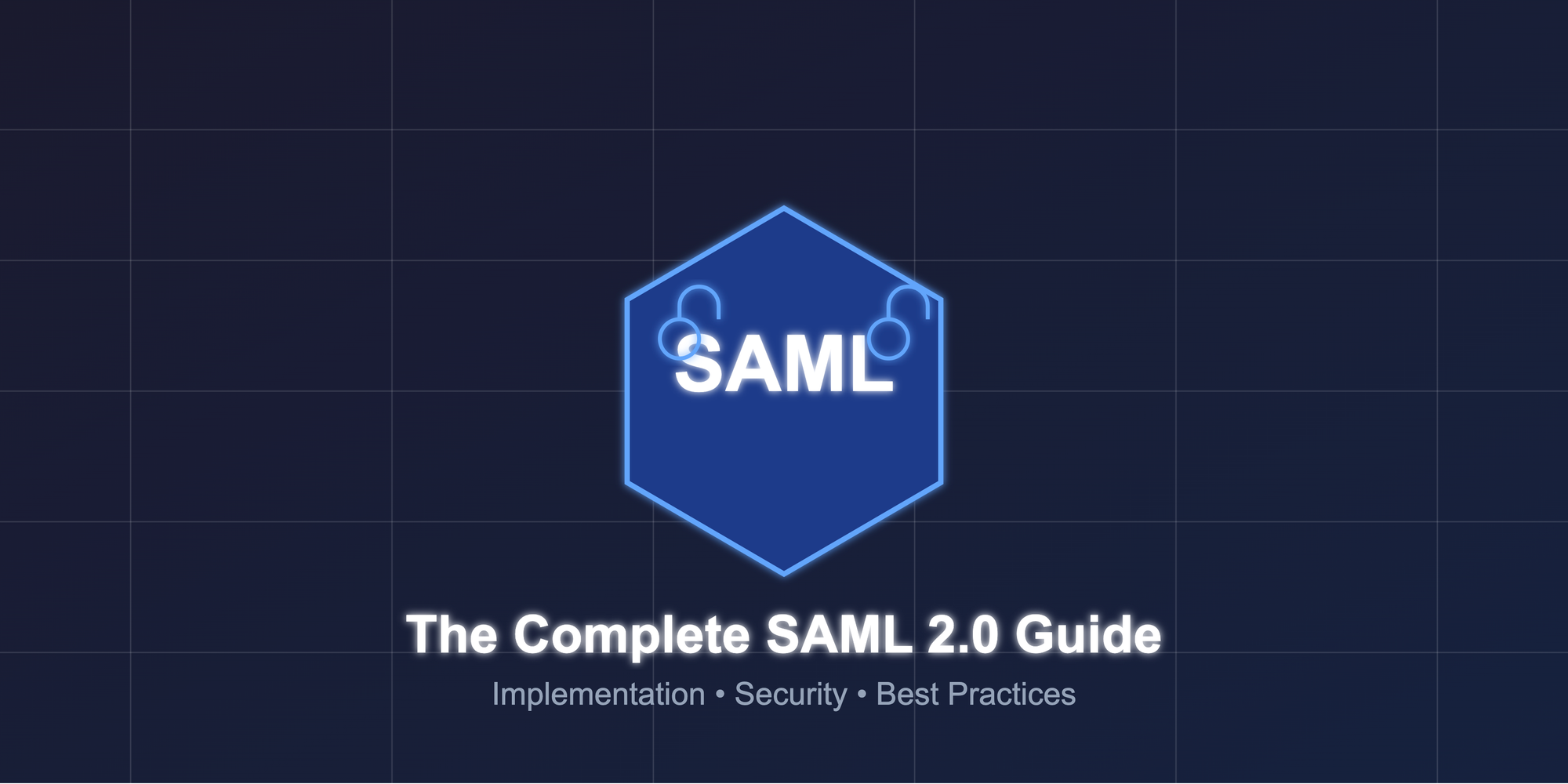
Security Assertion Markup Language (SAML) is an open standard for exchanging authentication and authorization data between parties, specifically between an identity provider (IdP) and a service provider (SP). Developed by the Security Services Technical Committee of OASIS (Organization for the Advancement of Structured Information Standards), SAML has become a cornerstone of modern identity and access management (IAM) systems.
Historical Context
SAML's journey began in the early 2000s when organizations started grappling with the challenge of securely sharing identity information across different security domains. The first version, SAML 1.0, was ratified in November 2002. It was followed by SAML 1.1 in September 2003, which introduced minor updates.
The real breakthrough came with SAML 2.0, released in March 2005. This version represented a significant overhaul, incorporating feedback from real-world implementations and merging with other standards like Shibboleth and ID-FF (Identity Federation Framework).
Importance in the IAM Landscape
SAML plays a crucial role in enabling single sign-on (SSO) across different domains, allowing users to access multiple applications with a single set of credentials. This not only enhances user experience but also strengthens security by reducing the number of credentials users need to manage.
Key benefits of SAML include:
- Improved user experience through SSO
- Enhanced security by centralizing authentication
- Reduced administrative overhead for managing user accounts
- Interoperability across different platforms and vendors
- Support for federated identity management
Here are the key areas, I'll cover in this article:
- Technical Background
- SAML Protocol Specification
- Implementation Guide
- Use Cases
- Integration with Other Systems
- Security Analysis
- Performance and Scalability
- Troubleshooting and Debugging
- Future Developments
- Comparison with Similar Protocols
- Regulatory Compliance
- Community and Resources
- Practical Exercises
- Glossary of Terms
- References and Further Reading
1. Technical Background
Foundational Concepts
To understand SAML, it's essential to grasp these key concepts:
- Assertions: These are the core of SAML. An assertion is a package of information that contains statements about a principal (usually a user).
- Protocols: SAML defines request/response protocols for obtaining assertions and for other identity management functions.
- Bindings: These define how SAML messages are mapped onto standard communication protocols like HTTP or SOAP.
- Profiles: SAML profiles describe how assertions, protocols, and bindings are combined to support a particular use case.
Related Standards
SAML doesn't exist in isolation. It's part of a broader ecosystem of identity standards:
- OAuth: While SAML focuses on enterprise SSO, OAuth is geared towards authorization, particularly for API access.
- OpenID Connect: Built on top of OAuth 2.0, it adds an identity layer, making it more comparable to SAML in some use cases.
- WS-Federation: Another standard for identity federation, often used in Microsoft environments.
Evolution of SAML
SAML has evolved significantly since its inception:
- SAML 1.0 (2002): Introduced the basic concepts of SAML assertions and protocols.
- SAML 1.1 (2003): Minor update, adding support for artifact bindings.
- SAML 2.0 (2005): Major revision, incorporating lessons from earlier versions and other standards. Introduced new features like metadata and identity provider discovery.
While SAML 2.0 remains the current version, the standard continues to evolve through extensions and profiles that address specific use cases or emerging technologies.
2. Protocol Specifications
Architecture
SAML's architecture is built around three key roles:
- Principal: The user who wants to access a service.
- Identity Provider (IdP): The system that authenticates the user and issues SAML assertions.
- Service Provider (SP): The system that consumes SAML assertions and provides the service the user wants to access.
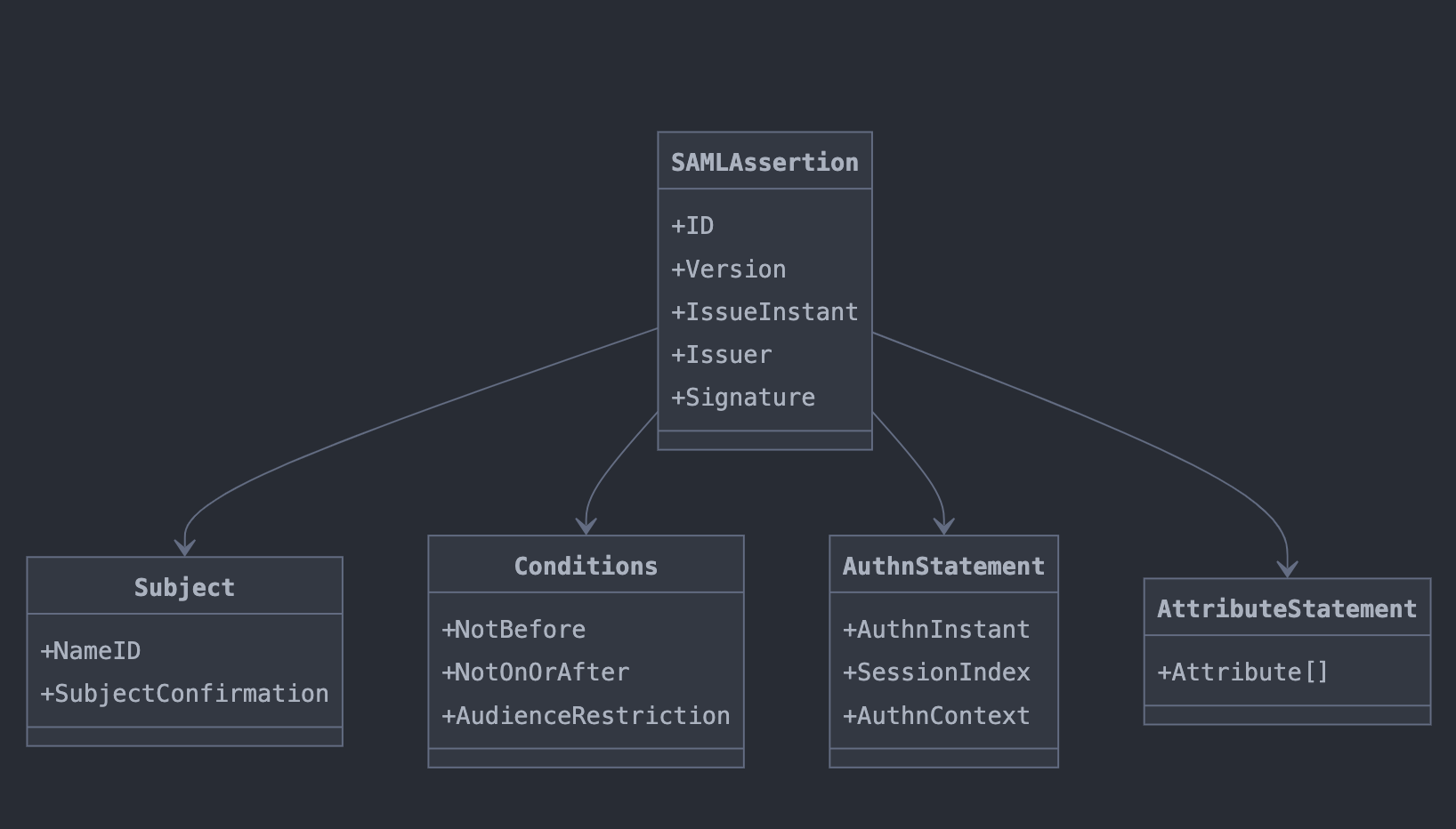
Core Components
- Assertions: These are XML-based statements about the principal. There are three types of assertions:
- Authentication assertions
- Attribute assertions
- Authorization decision assertions
- Protocols: SAML defines several request/response protocols, including:
- Authentication Request Protocol
- Single Logout Protocol
- Assertion Query and Request Protocol
- Artifact Resolution Protocol
- Name Identifier Management Protocol
- Bindings: These map SAML protocol messages to standard messaging or communication protocols. Common bindings include:
- HTTP Redirect Binding
- HTTP POST Binding
- HTTP Artifact Binding
- SAML SOAP Binding
- Profiles: These define how SAML assertions, protocols, and bindings combine to support use cases. The Web Browser SSO Profile is one of the most widely used.
Data Formats and Structures
SAML uses XML for its messages. Here's a simplified example of a SAML assertion:
<saml:Assertion
xmlns:saml="urn:oasis:names:tc:SAML:2.0:assertion"
Version="2.0"
IssueInstant="2023-04-01T19:25:14Z">
<saml:Issuer>https://idp.example.com</saml:Issuer>
<saml:Subject>
<saml:NameID>john.doe@example.com</saml:NameID>
</saml:Subject>
<saml:Conditions
NotBefore="2023-04-01T19:25:14Z"
NotOnOrAfter="2023-04-01T19:30:14Z"/>
<saml:AuthnStatement
AuthnInstant="2023-04-01T19:25:14Z">
<saml:AuthnContext>
<saml:AuthnContextClassRef>
urn:oasis:names:tc:SAML:2.0:ac:classes:Password
</saml:AuthnContextClassRef>
</saml:AuthnContext>
</saml:AuthnStatement>
</saml:Assertion>
Protocol Flow
A typical SAML Web Browser SSO flow proceeds as follows:
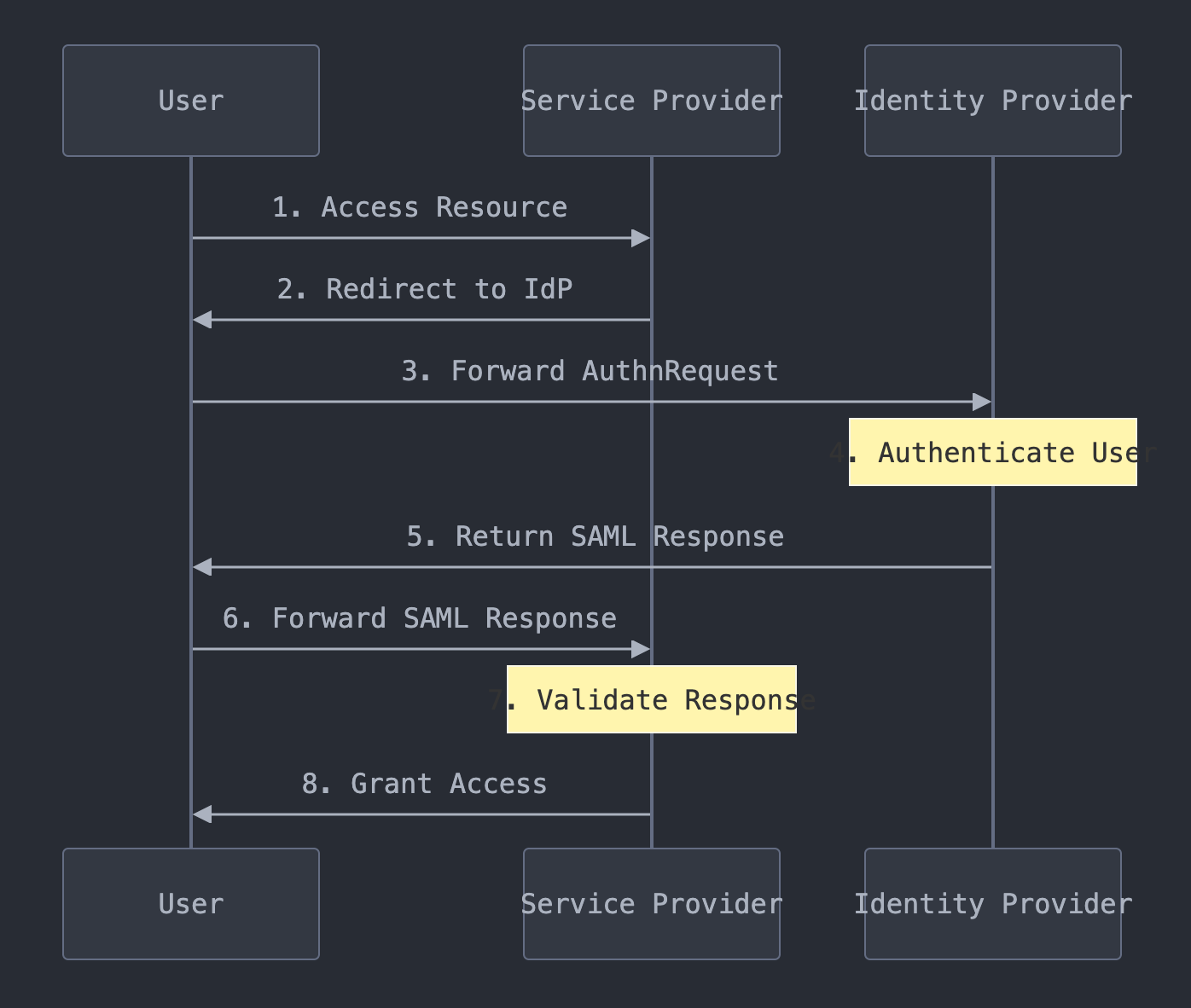
- User attempts to access a resource at the SP.
- SP checks if the user is already authenticated. If not, it generates a SAML authentication request.
- SP redirects the user's browser to the IdP with the SAML request.
- IdP authenticates the user (if not already authenticated).
- IdP generates a SAML response containing an assertion.
- IdP returns the SAML response to the user's browser.
- The browser posts the SAML response to the SP.
- SP validates the SAML response and extracts the assertion.
- If valid, SP grants access to the requested resource.
Security Considerations
SAML incorporates several security features:
- XML Signature for message integrity and authentication
- XML Encryption for confidentiality
- TLS/SSL for transport-level security
- Time-based validity periods for assertions
- Audience restrictions to prevent assertion misuse
3. Implementation Guide
Step-by-step Implementation
- Choose your tools: Select SAML libraries appropriate for your programming language (e.g., OpenSAML for Java, python3-saml for Python).
- Configure your environment: Set up your development environment with the necessary dependencies.
- Generate key pairs: Create public/private key pairs for signing and encrypting SAML messages.
- Implement SP functionality:
- Create endpoints for receiving SAML responses
- Implement SAML request generation
- Develop logic for validating and processing SAML responses
- Implement IdP functionality (if required):
- Set up user authentication mechanisms
- Implement SAML assertion generation
- Create endpoints for receiving and processing SAML requests
- Configure metadata: Create and exchange metadata with your identity provider or service providers.
- Implement error handling: Develop robust error handling for various SAML-related issues (e.g., invalid signatures, expired assertions).
- Test thoroughly: Use SAML testing tools to verify your implementation.
Code Example (Python with python3-saml)
Here's a simplified example of how to implement SP-initiated SSO using python3-saml:
from onelogin.saml2.auth import OneLogin_Saml2_Auth
from onelogin.saml2.settings import OneLogin_Saml2_Settings
def init_saml_auth(req):
saml_settings = OneLogin_Saml2_Settings(settings=None, custom_base_path=settings.SAML_FOLDER)
return OneLogin_Saml2_Auth(req, saml_settings)
def prepare_flask_request(request):
# Convert Flask request to SAML toolkit format
return {
'https': 'on' if request.scheme == 'https' else 'off',
'http_host': request.host,
'script_name': request.path,
'get_data': request.args.copy(),
'post_data': request.form.copy()
}
@app.route('/saml/login')
def saml_login():
req = prepare_flask_request(request)
auth = init_saml_auth(req)
return redirect(auth.login())
@app.route('/saml/acs', methods=['POST'])
def saml_acs():
req = prepare_flask_request(request)
auth = init_saml_auth(req)
auth.process_response()
errors = auth.get_errors()
if not errors:
if auth.is_authenticated():
# User is authenticated, set session and redirect
session['samlUserdata'] = auth.get_attributes()
return redirect(url_for('index'))
return "Authentication failed", 401
Best Practices
- Always validate SAML responses, including signatures and conditions.
- Use strong cryptographic algorithms for signing and encryption.
- Implement proper error handling and logging.
- Regularly update your SAML libraries to address security vulnerabilities.
- Use HTTPS for all SAML-related communications.
- Implement proper session management on the SP side.
- Regularly rotate signing and encryption keys.
Common Pitfalls
- Incorrectly configured clock synchronization between IdP and SP.
- Mismatched entity IDs or endpoints in metadata.
- Improper handling of signed vs. encrypted assertions.
- Neglecting to validate the entire response, not just the assertion.
- Failing to implement proper replay attack prevention.
4. Use Cases
SAML is widely used across various industries for different purposes:
- Enterprise SSO: Large organizations use SAML to provide seamless access to multiple internal applications.
- Cloud Service Access: SAML enables secure access to SaaS applications like Salesforce, Google Workspace, or Office 365.
- Higher Education: Many universities use SAML for federated access to academic resources and services.
- Healthcare: SAML facilitates secure sharing of patient information across different healthcare providers.
- Government: Many e-government services use SAML for citizen authentication and access control.
5. Integration with Other Systems
Compatibility with Other IAM Protocols
While SAML is powerful, it often needs to coexist with other protocols:
- OAuth 2.0 and OpenID Connect: Many organizations use SAML for web SSO and OAuth/OIDC for API access and mobile apps.
- WS-Federation: In Microsoft-heavy environments, SAML often needs to integrate with WS-Federation.
Integration with Identity Providers
SAML can integrate with various types of IdPs:
- On-premises IdPs: Products like Microsoft Active Directory Federation Services (ADFS) or Shibboleth.
- Cloud-based IdPs: Services like Okta, Azure AD, or Google Cloud Identity.
- Social IdPs: While not common, it's possible to bridge SAML with social login providers.
Interoperability Considerations
- Metadata Exchange: Ensure proper exchange and regular updates of SAML metadata between SP and IdP.
- Attribute Mapping: Coordinate attribute names and formats between SP and IdP.
- Authentication Context: Agree on supported authentication methods and their corresponding context classes.
- Binding Support: Ensure both SP and IdP support the same SAML bindings.
6. Security Analysis
Known Vulnerabilities
- XML Signature Wrapping Attacks: Attackers can sometimes manipulate the structure of SAML messages to bypass signature validation.
- Replay Attacks: If not properly mitigated, valid SAML assertions can be captured and reused.
- Man-in-the-Middle (MitM) Attacks: Especially when metadata or assertions are not properly secured.
- Malicious Identity Provider: In some federation scenarios, a compromised IdP can be a significant threat.
Mitigation Strategies
- Use XML Digital Signature Reference validation to prevent XML Signature Wrapping attacks.
- Implement proper replay prevention using the
NotBefore
andNotOnOrAfter
conditions, along with assertion ID tracking. - Always use HTTPS for SAML communications to prevent MitM attacks.
- Implement strict metadata validation and use out-of-band methods to verify IdP trustworthiness.
Security Best Practices
- Regularly update SAML libraries and dependencies.
- Use strong, unique keys for signing and encryption.
- Implement proper session management on the SP side.
- Use secure random number generators for all random values.
- Implement proper logging and monitoring for SAML transactions.
- Regularly conduct security audits and penetration testing.
Audit and Compliance Considerations
SAML implementations often need to comply with various standards and regulations:
- SOC 2: Ensure proper access controls and monitoring.
- GDPR: Implement appropriate data protection measures, especially for personal data in SAML assertions.
- HIPAA: In healthcare scenarios, ensure SAML implementations meet HIPAA security requirements.
- PCI DSS: For systems handling payment card data, ensure SAML meets relevant PCI DSS requirements.
Regular audits should be conducted to ensure ongoing compliance and security.
7. Performance and Scalability
Benchmarks and Performance Metrics
Key performance indicators for SAML implementations include:
- Response Time: The time taken to complete a full SAML authentication flow.
- Throughput: The number of SAML transactions that can be processed per unit of time.
- CPU and Memory Usage: Resource utilization during SAML processing.
Benchmark results will vary based on hardware, software, and specific implementations, but here are some general guidelines:
- SAML authentication flows should typically complete in under 2 seconds.
- High-performance SAML implementations should be able to handle thousands of authentications per minute.
- XML parsing and cryptographic operations are typically the most resource-intensive parts of SAML processing.
Scaling Strategies
- Load Balancing: Distribute SAML processing across multiple servers.
- Caching: Implement efficient caching of validated assertions and metadata.
- Asynchronous Processing: Use asynchronous I/O for SAML operations where possible.
- Hardware Security Modules (HSMs): Offload cryptographic operations to dedicated hardware.
- Optimize XML Processing: Use efficient XML parsing libraries and techniques.
Optimization Techniques
- Minimize SAML Assertion Size: Only include necessary attributes in assertions.
- Use Appropriate Bindings: HTTP-POST is generally more efficient than HTTP-Redirect for large SAML messages.
- Efficient Session Management: Implement efficient session storage and retrieval mechanisms.
- Metadata Caching: Cache and periodically refresh SAML metadata instead of fetching it for every transaction.
- Connection Pooling: Use connection pooling for database and LDAP connections related to SAML processing.
8. Troubleshooting and Debugging
Common Issues and Solutions
- "Invalid Signature" Errors
- Solution: Verify that the correct signing certificate is being used and that the entire SAML response is being validated, not just the assertion. Ensure the signature algorithm used matches what's expected.
- Clock Synchronization Issues
- Solution: Synchronize clocks between IdP and SP using NTP. Implement a small time tolerance (e.g., 5 minutes) to account for minor discrepancies.
- Metadata Mismatch
- Solution: Double-check that the metadata for both IdP and SP is up-to-date and correctly configured. Verify entity IDs, endpoints, and certificate information.
- Incorrect Binding Usage
- Solution: Ensure both IdP and SP are using compatible bindings (e.g., HTTP-POST, HTTP-Redirect) for each SAML message exchange.
- Missing or Incorrect Attributes
- Solution: Verify attribute mapping configuration on both IdP and SP sides. Ensure required attributes are being released by the IdP and correctly interpreted by the SP.
Debugging Tools and Techniques
- SAML Tracer: A browser extension that captures SAML messages exchanged between SP and IdP.
- XML Pretty Printers: Tools to format SAML XML for easier reading and analysis.
- Online SAML Decoders: Web services that decode and display the contents of SAML messages.
- Fiddler or Wireshark: Network analysis tools to capture and inspect SAML traffic.
- IdP-initiated Testing Tools: Many IdPs provide tools to initiate SAML flows for testing purposes.
Logging and Monitoring Best Practices
- Implement Comprehensive Logging:
- Log all SAML transactions, including requests, responses, and any validation errors.
- Include timestamps, session IDs, and user identifiers (taking care not to log sensitive information).
- Use Structured Logging:
- Adopt a structured logging format (e.g., JSON) for easier parsing and analysis.
- Implement Log Levels:
- Use different log levels (DEBUG, INFO, WARN, ERROR) to control logging verbosity.
- Monitor Key Metrics:
- Track authentication success/failure rates, response times, and error frequencies.
- Set Up Alerts:
- Configure alerts for unusual patterns, such as sudden increases in authentication failures or response time spikes.
- Regular Log Analysis:
- Perform regular reviews of SAML logs to identify potential issues or security concerns.
- Comply with Security Policies:
- Ensure logging practices comply with relevant security policies and regulations, particularly regarding sensitive data.
9. Future Developments
Upcoming Features or Versions
While SAML 2.0 has been stable for many years, the identity community continues to work on improvements:
- Enhanced Mobile Support: Efforts to make SAML more efficient for mobile applications.
- Improved Metadata Management: Tools and standards for more dynamic and automated metadata exchange.
- Integration with Emerging Standards: Work on better integration between SAML and newer standards like OpenID Connect.
Potential Improvements
- Simplified Configuration: Tools and standards to make SAML setup and configuration less complex.
- Performance Optimizations: Techniques to reduce the overhead of XML processing and cryptographic operations.
- Enhanced Privacy Features: Additional controls for attribute release and consent management.
Industry Trends Affecting SAML's Future
- Shift Towards OAuth and OpenID Connect: While SAML remains strong in enterprise environments, there's a trend towards OAuth and OIDC, especially for consumer-facing applications.
- Zero Trust Architecture: SAML's role in implementing zero trust security models.
- Passwordless Authentication: How SAML can adapt to support passwordless authentication methods.
- Blockchain and Decentralized Identity: Potential integration of SAML with blockchain-based identity systems.
10. Comparison with Similar Protocols
SAML vs. OAuth 2.0
Aspect | SAML | OAuth 2.0 |
---|---|---|
Primary Purpose | Authentication and Authorization | Authorization |
Message Format | XML | JSON |
Complexity | Higher | Lower |
Mobile/API Friendliness | Less Friendly | More Friendly |
Enterprise Adoption | High | Moderate |
SAML vs. OpenID Connect
Aspect | SAML | OpenID Connect |
---|---|---|
Built On | XML, SOAP | OAuth 2.0, JSON |
Complexity | Higher | Lower |
Mobile Support | Limited | Strong |
Discovery | IdP Discovery Profile | Dynamic Discovery |
Enterprise Features | Extensive | Growing |
When to Choose SAML
- For enterprise single sign-on scenarios
- When extensive identity federation features are required
- In environments with existing SAML infrastructure
- For integration with legacy systems
11. Regulatory Compliance
Relevant Standards and Regulations
- GDPR (General Data Protection Regulation):
- Ensure proper consent for attribute sharing
- Implement data minimization in SAML assertions
- Provide user control over shared attributes
- CCPA (California Consumer Privacy Act):
- Similar to GDPR, focus on user consent and data control
- Ensure proper disclosure of data sharing via SAML
- HIPAA (Health Insurance Portability and Accountability Act):
- Implement strong encryption for SAML assertions containing health information
- Ensure proper access controls and audit logging
- PCI DSS (Payment Card Industry Data Security Standard):
- If handling payment information, ensure SAML implementations meet relevant PCI DSS requirements
- Implement strong cryptography and key management
How SAML Helps Achieve Compliance
- Centralized Authentication: Simplifies access control and audit processes
- Attribute-based Access Control: Enables fine-grained access policies
- Encryption and Signing: Provides data integrity and confidentiality
- Single Logout: Helps ensure timely session termination across multiple services
12. Community and Resources
Open-source Projects and Tools
- OpenSAML: Java and C++ libraries for SAML
- python3-saml: Python toolkit for SAML
- SAML Toolkit: Available for various languages
- SimpleSAMLphp: PHP implementation of SAML
Official Documentation and Specifications
Community Forums and Support Channels
Training and Certification Options
- Shibboleth Consortium Training
- Master SAML2.0 with Okta
- SAML Security
- Vendor-specific certifications (e.g., Okta, Ping Identity)
13. Practical Exercises
- Setting up a Basic SAML SP:
- Implement a simple service provider using your chosen programming language and SAML library
- Configure it to work with a public SAML IdP sandbox
- Implementing SAML SSO in a Web Application:
- Add SAML-based authentication to an existing web application
- Handle user attributes and implement authorization based on SAML assertions
- SAML Metadata Management:
- Create and exchange SAML metadata between an SP and IdP
- Implement dynamic metadata refresh
- Troubleshooting SAML Issues:
- Set up deliberately misconfigured SAML connections and practice diagnosing and fixing issues
14. Glossary of Terms
- Assertion: A package of information that supplies one or more statements made by a SAML authority
- Binding: Defines how SAML protocol messages are communicated
- Identity Provider (IdP): The system that authenticates a user and issues SAML assertions
- Service Provider (SP): The system that consumes SAML assertions to authenticate and authorize users
- Metadata: Configuration information for SAML entities, typically in XML format
- Profile: A set of rules describing how to embed assertions into and extract them from a protocol or framework
- Single Sign-On (SSO): The ability for a user to authenticate once and gain access to multiple systems
- Single Logout (SLO): The ability to terminate all sessions created via SAML SSO simultaneously
15. References and Further Reading
- Hughes, J., et al. (2005). "Profiles for the OASIS Security Assertion Markup Language (SAML) V2.0"
- Ragouzis, N., et al. (2008). "Security Assertion Markup Language (SAML) V2.0 Technical Overview"
- Gross, T., et al. (2005). "A Security Analysis of SAML Single Sign-on Browser Artifact Profile"
- "SAML 2.0 Specification Errata" - OASIS Committee Specification
- "Secure Identity Management with SAML" - O'Reilly Media
- "Solving Identity Management in Modern Applications: Demystifying OAuth 2.0, OpenID Connect, and SAML 2.0" - Apress