Mastering Efficient Data Processing for LLMs, Generative AI, and Semantic Search
Discover cutting-edge techniques for optimizing data processing in LLMs, generative AI, and semantic search. Learn to leverage vector databases, implement data compression, utilize parallelization, and employ strategic caching
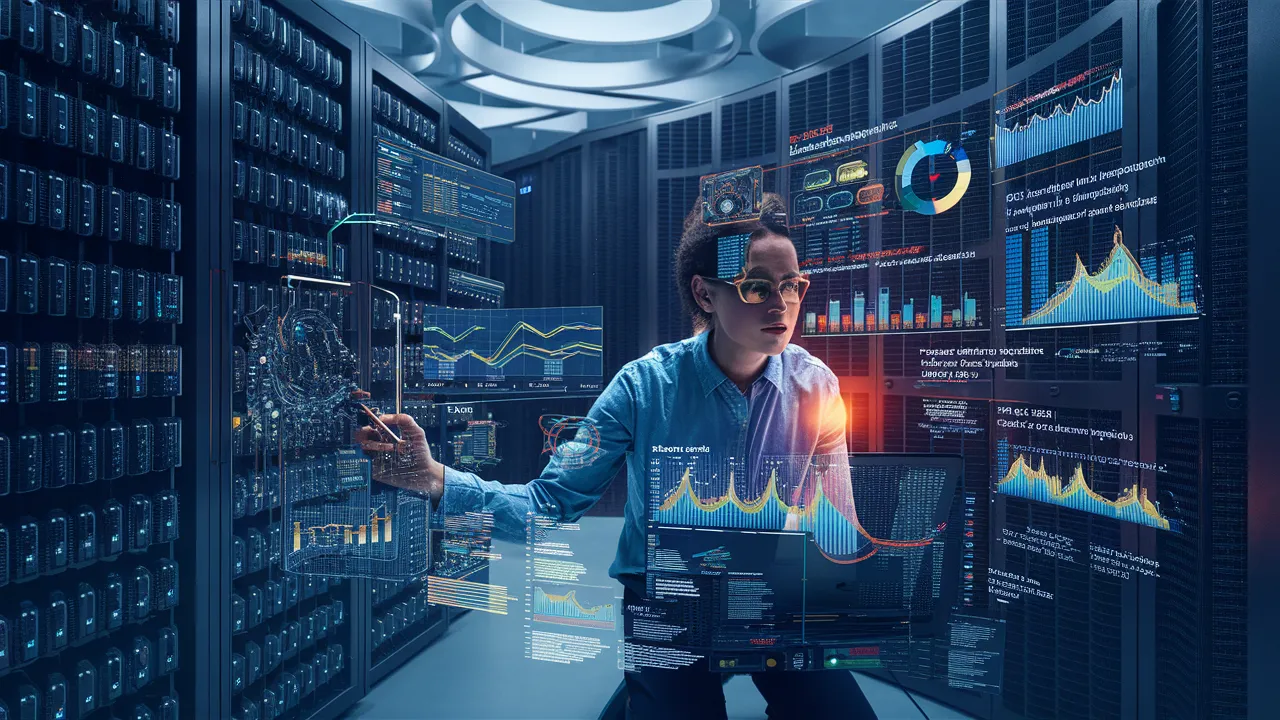
In modern AI applications, efficient data processing isn't just a desirable feature—it's a necessity. These advanced AI applications are reshaping industries, from natural language processing to content creation and information retrieval. Large Language Models (LLMs), generative AI models, and semantic search engines are all data-hungry beasts, and their performance is heavily reliant on the speed and efficiency with which they can access and process information.
However, their power comes at a cost: the need for efficient and scalable data processing pipelines. In this comprehensive guide, we'll explore cutting-edge techniques and strategies to optimize data processing for these AI-powered applications, focusing on leveraging vector databases, data compression, parallelization, and caching.
Understanding the Data Processing Challenges
Before diving into optimization techniques, it's crucial to understand the unique challenges posed by LLMs, generative AI, and semantic search:
a) Massive Data Volumes: LLMs are trained on enormous datasets, often comprising hundreds of gigabytes or even terabytes of text.
b) High-Dimensional Embeddings: Semantic search and many LLM applications rely on high-dimensional vector representations of text, which can be computationally expensive to process and store.
c) Real-time Requirements: Many applications, especially in semantic search, require near-instantaneous responses, putting pressure on processing pipelines.
d) Continuous Learning: Some systems need to update their knowledge base in real-time, necessitating efficient incremental processing.
Key Strategies for Efficient Data Processing
- Vector Databases
- Data Compression
- Parallel Processing
- Caching
- Hardware Acceleration
- Optimize Algorithms
- Data Cleaning and Preprocessing
- Continuous Optimization
- Optimizing for Specific Use Case
1. Leveraging Vector Databases
Vector databases have emerged as a crucial tool for managing high-dimensional embeddings efficiently. Here's how to make the most of them:
a) Choosing the Right Vector Database
- FAISS (Facebook AI Similarity Search): Excellent for large-scale similarity search and clustering.
- Milvus: An open-source vector database with strong scalability and ease of use.
- Pinecone: A fully managed vector database service with advanced features like hybrid search.
b) Indexing Strategies
- Implement Approximate Nearest Neighbor (ANN) algorithms like HNSW (Hierarchical Navigable Small World) for faster similarity search.
- Use Product Quantization (PQ) to compress vectors while maintaining search quality.
c) Sharding and Distributed Processing
- Implement horizontal sharding to distribute vector data across multiple nodes.
- Use consistent hashing for efficient data distribution and retrieval.
Example Python code snippet using FAISS for efficient similarity search:
import faiss
import numpy as np
# Assume we have a set of embeddings
embeddings = np.random.random((100000, 128)).astype('float32')
# Create an index
index = faiss.IndexFlatL2(128)
# Add vectors to the index
index.add(embeddings)
# Perform a search
query = np.random.random((1, 128)).astype('float32')
k = 5 # number of nearest neighbors
D, I = index.search(query, k)
print(f"Distances: {D}")
print(f"Indices: {I}")
2. Data Compression Techniques
Efficient data compression is vital for managing large datasets and reducing storage and transmission costs:
a) Quantization
- Scalar quantization: Reduce the precision of floating-point numbers.
- Vector quantization: Represent groups of vectors with a smaller set of centroids.
b) Dimensionality Reduction
- Principal Component Analysis (PCA): Reduce the dimensionality of embeddings while preserving most of the information.
- Random Projection: A computationally efficient alternative to PCA for high-dimensional data.
c) Sparse Encoding
- Implement sparse representations for text data, such as bag-of-words or TF-IDF.
Example of dimensionality reduction using PCA:
from sklearn.decomposition import PCA
import numpy as np
# Assume we have high-dimensional embeddings
embeddings = np.random.random((10000, 768))
# Initialize PCA
pca = PCA(n_components=128)
# Fit and transform the data
reduced_embeddings = pca.fit_transform(embeddings)
print(f"Original shape: {embeddings.shape}")
print(f"Reduced shape: {reduced_embeddings.shape}")
3. Parallel Processing
Leveraging parallel processing can significantly speed up data processing pipelines:
a) Data Parallelism
- Distribute data across multiple nodes or GPUs for parallel processing.
- Implement map-reduce paradigms for large-scale data processing.
b) Model Parallelism
- For large LLMs, distribute different layers of the model across multiple GPUs.
c) Pipeline Parallelism
- Implement a pipeline where different stages of processing occur simultaneously on different data batches.
Example using Python's multiprocessing for parallel data processing:
from multiprocessing import Pool
import numpy as np
def process_chunk(chunk):
# Assume this is a computationally intensive operation
return np.mean(chunk, axis=0)
# Create a large dataset
data = np.random.random((1000000, 100))
# Split the data into chunks
chunks = np.array_split(data, 10)
# Process in parallel
with Pool(processes=4) as pool:
results = pool.map(process_chunk, chunks)
# Combine results
final_result = np.mean(results, axis=0)
4. Caching
Implementing effective caching can dramatically reduce computation time for frequently accessed data:
a) In-memory Caching
- Use libraries like Redis or Memcached for fast, in-memory caching of frequently accessed embeddings or search results.
b) Disk-based Caching
- Implement LRU (Least Recently Used) caching for larger datasets that don't fit in memory.
c) Predictive Caching
- Use machine learning models to predict and pre-cache likely queries or data accesses.
Example of implementing a simple LRU cache:
from functools import lru_cache
@lru_cache(maxsize=1000)
def compute_embedding(text):
# Assume this is a computationally expensive operation
# In reality, this would involve calling an LLM or embedding model
return hash(text)
# First call will compute the embedding
result1 = compute_embedding("Hello, world!")
# Second call will retrieve from cache
result2 = compute_embedding("Hello, world!")
print(f"Result 1: {result1}")
print(f"Result 2: {result2}")
5. Hardware Acceleration
Leveraging specialized hardware can dramatically improve processing speed and efficiency:
a) GPU Acceleration
- Utilize NVIDIA GPUs with CUDA for parallel processing of large matrices and vectors.
- Implement libraries like cuBLAS for GPU-accelerated linear algebra operations.
b) TPU (Tensor Processing Units)
- For large-scale deployments, consider using Google's TPUs, which are specifically designed for machine learning workloads.
c) FPGA (Field-Programmable Gate Arrays)
- Implement custom hardware accelerators for specific, repetitive tasks in your pipeline.
Example of using GPU acceleration with PyTorch:
import torch
# Check if CUDA is available
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Using device: {device}")
# Create a large tensor
x = torch.randn(10000, 10000, device=device)
# Perform a matrix multiplication
result = torch.matmul(x, x.t())
print(f"Result shape: {result.shape}")
6. Optimize Algorithms
Implementing efficient algorithms can significantly reduce computational complexity:
a) Approximate Nearest Neighbor (ANN) Algorithms
- Use algorithms like HNSW (Hierarchical Navigable Small World) or NSG (Navigable Spreading-out Graph) for faster similarity search.
b) Efficient Tokenization
- Implement BPE (Byte Pair Encoding) or SentencePiece for faster and more efficient tokenization of text data.
c) Pruning Techniques
- For LLMs, implement model pruning techniques to reduce model size without significant loss in performance.
Example of using HNSW for approximate nearest neighbor search:
import hnswlib
import numpy as np
# Generate sample data
dim = 128
num_elements = 100000
# Generating sample data
data = np.random.rand(num_elements, dim).astype('float32')
# Declaring index
p = hnswlib.Index(space='l2', dim=dim)
# Initializing index
p.init_index(max_elements=num_elements, ef_construction=200, M=16)
# Adding data points
p.add_items(data)
# Searching
k = 3
query_data = np.random.rand(1, dim).astype('float32')
labels, distances = p.knn_query(query_data, k=k)
print(f"Labels of {k} nearest neighbors: {labels}")
print(f"Distances to {k} nearest neighbors: {distances}")
7. Data Cleaning and Preprocessing
Effective data preparation is crucial for optimal performance:
a) Text Normalization
- Implement Unicode normalization, lowercasing, and special character handling.
b) Deduplication
- Remove duplicate or near-duplicate entries to reduce data size and improve model quality.
c) Intelligent Sampling
- For very large datasets, implement stratified sampling to maintain data distribution while reducing size.
Example of text preprocessing using Python:
import re
import unicodedata
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
def preprocess_text(text):
# Lowercase the text
text = text.lower()
# Normalize Unicode characters
text = unicodedata.normalize('NFKD', text).encode('ascii', 'ignore').decode('utf-8')
# Remove special characters and digits
text = re.sub(r'[^a-zA-Z\s]', '', text)
# Tokenize the text
tokens = word_tokenize(text)
# Remove stopwords
stop_words = set(stopwords.words('english'))
tokens = [token for token in tokens if token not in stop_words]
return ' '.join(tokens)
# Example usage
raw_text = "Hello, world! This is an example of text preprocessing. 123 @#$%"
processed_text = preprocess_text(raw_text)
print(f"Processed text: {processed_text}")
8. Continuous Optimization
Implement systems for ongoing performance improvement:
a) A/B Testing
- Continuously test different processing strategies and model configurations.
b) Automated Hyperparameter Tuning
- Use libraries like Optuna or Ray Tune for automated optimization of processing parameters.
c) Performance Monitoring
- Implement comprehensive logging and monitoring to identify bottlenecks and optimization opportunities.
Example of hyperparameter tuning with Optuna:
import optuna
def objective(trial):
# Define the hyperparameters to optimize
n_estimators = trial.suggest_int('n_estimators', 100, 1000)
max_depth = trial.suggest_int('max_depth', 1, 30)
min_samples_split = trial.suggest_int('min_samples_split', 2, 100)
# Create and train your model with these hyperparameters
model = RandomForestClassifier(n_estimators=n_estimators,
max_depth=max_depth,
min_samples_split=min_samples_split)
model.fit(X_train, y_train)
# Return the metric to optimize
return model.score(X_test, y_test)
# Create a study object and optimize the objective function
study = optuna.create_study(direction='maximize')
study.optimize(objective, n_trials=100)
print('Number of finished trials:', len(study.trials))
print('Best trial:')
trial = study.best_trial
print(' Value: ', trial.value)
print(' Params: ')
for key, value in trial.params.items():
print(' {}: {}'.format(key, value))
9. Optimizing for Specific Use Case
a) LLMs
- Implement efficient tokenization and batching strategies.
- Use quantization techniques to reduce model size and inference time.
b) Generative AI
- Implement beam search with early stopping for faster text generation.
- Use caching for partial results in iterative generation processes.
c) Semantic Search
- Implement hybrid search combining vector similarity with traditional keyword-based methods.
- Use hierarchical clustering for efficient search space pruning.
Conclusion
Mastering efficient data processing for LLMs, generative AI, and semantic search requires a multifaceted approach. By implementing advanced techniques such as vector databases, data compression, parallelization, and caching, and complementing them with hardware acceleration, optimized algorithms, thorough data preprocessing, and continuous optimization, you can create highly efficient and scalable AI-powered applications.
The key to success lies not just in implementing these strategies individually, but in finding the right balance and combination that works for your specific use case. Continuous monitoring, testing, and optimization are crucial in this rapidly evolving field.
As AI technologies continue to advance, staying informed about the latest developments in data processing techniques will be essential. By leveraging these cutting-edge strategies, you can push the boundaries of what's possible with AI, creating applications that are not only powerful and innovative but also efficient and responsive.
Remember, the goal is not just to process data faster, but to do so in a way that enables new possibilities and insights. With these advanced techniques at your disposal, you're well-equipped to tackle the challenges of building next-generation AI applications.